代码实现(C语言实现随机产生一个以三元组顺序表存储的稀疏矩阵,并对其进行转置)
时间: 2024-05-14 14:19:35 浏览: 13
好的,下面是实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100 // 稀疏矩阵最大元素个数
// 三元组结构体
typedef struct {
int i, j;
int value;
} Triple;
// 稀疏矩阵结构体
typedef struct {
Triple data[MAXSIZE + 1];
int rows, cols, nums;
} SparseMatrix;
// 初始化稀疏矩阵
void initSparseMatrix(SparseMatrix *matrix, int rows, int cols, int nums) {
matrix->rows = rows;
matrix->cols = cols;
matrix->nums = nums;
for (int i = 1; i <= nums; i++) {
scanf("%d%d%d", &matrix->data[i].i, &matrix->data[i].j, &matrix->data[i].value);
}
}
// 转置稀疏矩阵
SparseMatrix transpose(SparseMatrix matrix) {
SparseMatrix transposedMatrix;
transposedMatrix.rows = matrix.cols;
transposedMatrix.cols = matrix.rows;
transposedMatrix.nums = matrix.nums;
if (transposedMatrix.nums > 0) {
int q = 1;
for (int col = 1; col <= matrix.cols; col++) {
for (int p = 1; p <= matrix.nums; p++) {
if (matrix.data[p].j == col) {
transposedMatrix.data[q].i = matrix.data[p].j;
transposedMatrix.data[q].j = matrix.data[p].i;
transposedMatrix.data[q].value = matrix.data[p].value;
q++;
}
}
}
}
return transposedMatrix;
}
// 打印稀疏矩阵
void printSparseMatrix(SparseMatrix matrix) {
printf("rows = %d, cols = %d, nums = %d\n", matrix.rows, matrix.cols, matrix.nums);
for (int i = 1; i <= matrix.nums; i++) {
printf("(%d, %d, %d)\n", matrix.data[i].i, matrix.data[i].j, matrix.data[i].value);
}
}
int main() {
SparseMatrix matrix, transposedMatrix;
int rows, cols, nums;
printf("请输入稀疏矩阵的行数、列数和元素个数:\n");
scanf("%d%d%d", &rows, &cols, &nums);
printf("请输入稀疏矩阵的三元组:\n");
initSparseMatrix(&matrix, rows, cols, nums);
printf("稀疏矩阵为:\n");
printSparseMatrix(matrix);
transposedMatrix = transpose(matrix);
printf("转置后的稀疏矩阵为:\n");
printSparseMatrix(transposedMatrix);
return 0;
}
```
输入数据时,先输入稀疏矩阵的行数、列数和元素个数,然后输入矩阵的三元组。输出时先打印原稀疏矩阵,再打印转置后的稀疏矩阵。
请注意,这里的转置是指将稀疏矩阵的行与列互换,而不是将稀疏矩阵中的元素按照列主序重新排列。如果需要进行列主序的转置,需要修改转置函数。
相关推荐
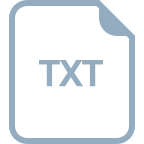
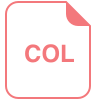
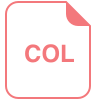
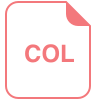
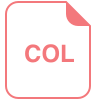
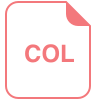









