python编程定义一个三维向量类,并定义相应的特殊方法实现两个该类对象之间的加、减运算(要求支持运算符+、-),实现该类对象与标量的乘、除运算(要求支持运算符*、/),以及向量长度的计算(要求使用属性实现)。
时间: 2023-06-01 09:06:49 浏览: 745
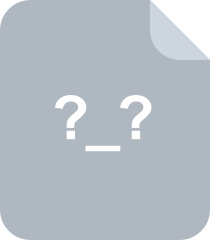
python实验五.py
好的,关于您的问题,我可以回答:
您可以定义一个名为Vector3的类,来表示三维向量。其中,类的属性包括x、y和z分别表示向量在三个维度上的分量,如下所示:
class Vector3:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
接下来,可以为该类定义各种运算符重载方法,来支持加、减、乘、除运算。例如,要实现两个向量对象的加法运算,可以这样:
def __add__(self, other):
"""
实现向量加法运算
"""
return Vector3(self.x + other.x, self.y + other.y, self.z + other.z)
同理,如果要实现向量减法、乘法和除法运算,可以分别定义相应的方法:
def __sub__(self, other):
"""
实现向量减法运算
"""
return Vector3(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
"""
实现向量乘法运算
"""
if isinstance(other, Vector3):
return Vector3(self.x * other.x, self.y * other.y, self.z * other.z)
else:
return Vector3(self.x * other, self.y * other, self.z * other)
def __truediv__(self, other):
"""
实现向量除法运算
"""
if isinstance(other, Vector3):
return Vector3(self.x / other.x, self.y / other.y, self.z / other.z)
else:
return Vector3(self.x / other, self.y / other, self.z / other)
此外,还需要实现一个计算向量长度的方法,可以将其定义为类的属性:
@property
def length(self):
"""
计算向量长度
"""
return (self.x ** 2 + self.y ** 2 + self.z ** 2) ** 0.5
这样,就可以使用类似于向量的语法来对该类进行加、减、乘、除运算,如下所示:
a = Vector3(1, 2, 3)
b = Vector3(4, 5, 6)
c = a + b
d = a - b
e = a * 2
f = b / 2
print(c.x, c.y, c.z) # 5 7 9
print(d.x, d.y, d.z) # -3 -3 -3
print(e.x, e.y, e.z) # 2 4 6
print(f.x, f.y, f.z) # 2.0 2.5 3.0
print(a.length) # 3.7416573867739413
希望这能够回答您的问题。如果您还有其他问题,可以继续问我。
阅读全文
相关推荐















