用python定义一个三维向量类,并定义相应的特殊方法实现两个该类对象之间的加、减运算(要求支持运算符+、-),实现该类对象与标量的乘、除运算(要求支持运算符*、/),以及向量长度的计算(要求使用属性实现)。
时间: 2023-06-09 18:04:01 浏览: 215
好的,我明白了。以下是用 Python 定义一个三维向量类及实现相应的特殊方法的代码:
```python
class Vector3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.y * other.z - other.y * self.z, self.z * other.x - other.z * self.x, self.x * other.y - other.x * self.y)
else:
return Vector3D(self.x * other, self.y * other, self.z * other)
def __truediv__(self, other):
return Vector3D(self.x / other, self.y / other, self.z / other)
@property
def length(self):
return (self.x ** 2 + self.y ** 2 + self.z ** 2) ** 0.5
```
在这个类中,我们定义了一个 `__init__` 方法以初始化三个参数,即向量的三个坐标(x,y,z)。我们还定义了相应的特殊方法,包括加、减、乘、除以及向量长度的计算方法。
注意,向量的乘法运算在这里我们采用了叉积运算的形式,如果要进行点积运算,可以改变 `__mul__` 方法的实现即可。
使用该类的实例可以像下面这样:
```python
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
v3 = v1 + v2
v4 = v1 - v2
v5 = v1 * v2
v6 = v1 / 2
l = v1.length
print(v3.x, v3.y, v3.z) # 5, 7, 9
print(v4.x, v4.y, v4.z) # -3, -3, -3
print(v5.x, v5.y, v5.z) # -3, 6, -3
print(v6.x, v6.y, v6.z) # 0.5, 1.0, 1.5
print(l) # 3.7416573867739413
```
在这里,我们定义了两个向量 `v1` 和 `v2`,然后进行了加、减、乘、除以及向量长度的计算,并将结果进行输出。
希望能帮到你!
阅读全文
相关推荐
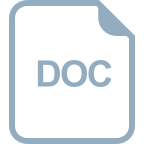
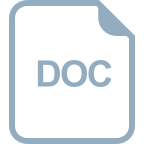
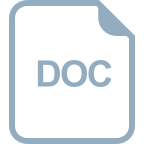











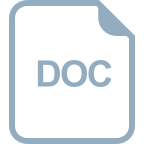
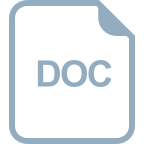
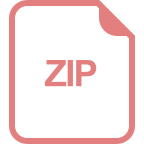
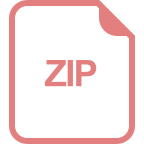