定义一个三维向量类,并定义相应的特殊方法实现:1)两个该类对象的加、减运算(要求支持运算符+、-);2)实现该类对象与标量的乘、除运算(要求支持运算符*、/,乘法时包括标量在前和后两种情况);3)以及向量的print方法; 向量的长度的定义;向量的点积以及叉积的定义; 定义一个类属性,表征已经实例化的对象的个数。
时间: 2023-10-27 16:30:33 浏览: 135
好的,以下是三维向量类的代码实现:
```python
class Vector3D:
count = 0 # 类属性,表征已经实例化的对象的个数
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
Vector3D.count += 1
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
if isinstance(other, (int, float)):
return Vector3D(self.x * other, self.y * other, self.z * other)
elif isinstance(other, Vector3D):
return self.x * other.x + self.y * other.y + self.z * other.z
def __rmul__(self, other):
return Vector3D(self.x * other, self.y * other, self.z * other)
def __truediv__(self, other):
return Vector3D(self.x / other, self.y / other, self.z / other)
def print(self):
print(f"<{self.x}, {self.y}, {self.z}>")
def length(self):
return (self.x ** 2 + self.y ** 2 + self.z ** 2) ** 0.5
def dot_product(self, other):
return self.x * other.x + self.y * other.y + self.z * other.z
def cross_product(self, other):
x = self.y * other.z - self.z * other.y
y = self.z * other.x - self.x * other.z
z = self.x * other.y - self.y * other.x
return Vector3D(x, y, z)
```
其中,`__add__`和`__sub__`方法分别实现了向量的加、减运算,`__mul__`和`__rmul__`方法实现了向量与标量的乘法运算,`__truediv__`方法实现了向量与标量的除法运算。
`print`方法实现向量的输出,`length`方法实现向量的长度计算,`dot_product`方法实现向量的点积计算,`cross_product`方法实现向量的叉积计算。
类属性`count`记录已经实例化的对象的个数。
可以通过以下代码进行测试:
```python
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(-1, 4, 2)
# 加减运算
v3 = v1 + v2
v4 = v1 - v2
v3.print() # 输出 <0, 6, 5>
v4.print() # 输出 <2, -2, 1>
# 乘除运算
v5 = v1 * 2
v6 = 3 * v2
v7 = v1 / 2
v5.print() # 输出 <2, 4, 6>
v6.print() # 输出 <-3, 12, 6>
v7.print() # 输出 <0.5, 1.0, 1.5>
# 长度计算
print(v1.length()) # 输出 3.7416573867739413
# 点积计算
print(v1.dot_product(v2)) # 输出 12
# 叉积计算
v8 = v1.cross_product(v2)
v8.print() # 输出 <-10, -5, 6>
# 对象个数
print(Vector3D.count) # 输出 2
```
阅读全文
相关推荐
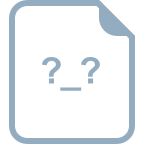
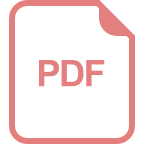











