Python 定义一个三维向量类,并定义相应的特殊方法实现两个该类对象之间的加、减运算,实现该类对象与标量的乘、除运算,以及向量长度的计算(要求使用属性实现)。
时间: 2024-10-24 17:02:46 浏览: 42
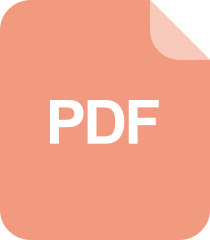
python实现比较类的两个instance(对象)是否相等的方法分析
在Python中,我们可以定义一个名为`Vector3D`的类,用于表示三维向量,并通过继承`object`并实现特殊方法(也称为魔术方法)来支持所需的数学操作。这里是一个简单的实现:
```python
class Vector3D(object):
def __init__(self, x=0, y=0, z=0):
self.x = x
self.y = y
self.z = z
# 特殊方法:相加
def __add__(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
elif isinstance(other, (int, float)):
return Vector3D(self.x + other, self.y + other, self.z + other)
else:
raise TypeError("Unsupported operand type(s) for +: 'Vector3D' and '{}'".format(type(other)))
# 特殊方法:相减
def __sub__(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
elif isinstance(other, (int, float)):
return Vector3D(self.x - other, self.y - other, self.z - other)
else:
raise TypeError("Unsupported operand type(s) for -: 'Vector3D' and '{}'".format(type(other)))
# 特殊方法:与标量乘法
def __mul__(self, scalar):
if isinstance(scalar, (int, float)):
return Vector3D(self.x * scalar, self.y * scalar, self.z * scalar)
else:
raise TypeError("Unsupported operand type(s) for *: 'Vector3D' and '{}'".format(type(scalar)))
# 特殊方法:与标量除法
def __truediv__(self, scalar):
if isinstance(scalar, (int, float)) and scalar != 0:
return Vector3D(self.x / scalar, self.y / scalar, self.z / scalar)
else:
raise ZeroDivisionError("Cannot divide by zero")
# 特殊方法:向量长度(欧几里得距离)
def __len__(self):
return (self.x**2 + self.y**2 + self.z**2)**0.5
# 示例
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
print("v1 + v2 =", v1 + v2)
print("v1 - v2 =", v1 - v2)
print("v1 * 2 =", v1 * 2)
print("v1 / 2 =", v1 / 2) # 注意这里会抛出异常,因为不能对非零整数做除法
print("Length of v1 =", len(v1))
```
阅读全文