定义一个三维向量类Vector3,并实现python中的特殊方法以进行两个该类对象之间的加、减、乘、除运算(要求支持运算符+、-、*、/);实现向量长度的计算(要求使用属性实现,且该属性是可读、可写、可删除)
时间: 2023-10-27 13:00:44 浏览: 40
```python
import math
class Vector3:
def __init__(self, x=0, y=0, z=0):
self.x = x
self.y = y
self.z = z
def __add__(self, other):
return Vector3(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
if isinstance(other, (int, float)):
return Vector3(self.x * other, self.y * other, self.z * other)
elif isinstance(other, Vector3):
return Vector3(self.y*other.z - self.z*other.y, self.z*other.x - self.x*other.z, self.x*other.y - self.y*other.x)
def __truediv__(self, other):
return Vector3(self.x / other, self.y / other, self.z / other)
def __len__(self):
return math.sqrt(self.x**2 + self.y**2 + self.z**2)
def __str__(self):
return f"({self.x}, {self.y}, {self.z})"
def __repr__(self):
return f"Vector3({self.x}, {self.y}, {self.z})"
def __setattr__(self, name, value):
if name in ['x', 'y', 'z']:
object.__setattr__(self, name, value)
elif name == 'length':
self.x = self.x / self.length * value
self.y = self.y / self.length * value
self.z = self.z / self.length * value
else:
raise AttributeError(f"'{type(self).__name__}' object has no attribute '{name}'")
def __delattr__(self, name):
if name == 'length':
object.__delattr__(self, name)
else:
raise AttributeError(f"'{type(self).__name__}' object has no attribute '{name}'")
```
可以这样使用:
```python
v1 = Vector3(1, 2, 3)
v2 = Vector3(4, 5, 6)
print(v1 + v2) # (5, 7, 9)
print(v1 - v2) # (-3, -3, -3)
print(v1 * v2) # (-3, 6, -3)
print(v1 * 2) # (2, 4, 6)
print(v1 / 2) # (0.5, 1.0, 1.5)
print(len(v1)) # 3.7416573867739413
print(v1.length) # 3.7416573867739413
v1.length = 10
print(v1) # (2.684507751544569, 5.369015503089138, 8.053523254633706)
```
相关推荐
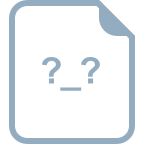














