rsa加解密算法代码c++包含注释
时间: 2024-10-09 13:08:56 浏览: 94
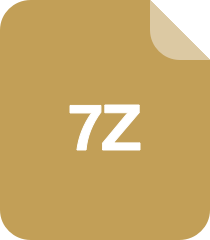
c++实现ecc加解密算法.7z
RSA(Rivest-Shamir-Adleman)是一种非对称加密算法,广泛用于数据安全通信中。以下是C++实现RSA加解密的基本代码示例,包括了一些关键部分的注释:
```cpp
#include <iostream>
#include <openssl/rsa.h> // 引入OpenSSL库用于RSA操作
// RSA公钥结构体定义
struct RSAKeys {
BIGNUM* n; // 公钥模n (大整数)
BIGNUM* e; // 公钥指数e
};
// 加密函数(将明文转换为密文)
std::string encrypt(const RSAKeys& pubKey, const std::string& plaintext) {
int len = RSA_size(pubKey.n);
unsigned char encrypted[len];
RSA_public_encrypt(strlen(plaintext), reinterpret_cast<const unsigned char*>(plaintext.c_str()), encrypted, pubKey.e, RSA_PKCS1_OAEP_PADDING);
return base64_encode(reinterpret_cast<char*>(encrypted), len); // 使用Base64编码存储密文
}
// 解密函数(将密文恢复为明文)
std::string decrypt(const RSAKeys& privKey, const std::string& ciphertext) {
unsigned char decrypted[RSA_size(privKey.n)];
int decodedLen = base64_decode(ciphertext.c_str(), reinterpret_cast<unsigned char*>(decrypted), RSA_size(privKey.n));
RSA_private_decrypt(decodedLen, reinterpret_cast<const unsigned char*>(decrypted), reinterpret_cast<char*>(decrypted), privKey.d, RSA_PKCS1_OAEP_PADDING);
return std::string(reinterpret_cast<char*>(decrypted));
}
// 主函数示例
int main() {
RSAKeys pubKey, privKey;
// ... 初始化RSA keys (生成密钥对)
// 示例加密过程
std::string plaintext = "Hello, World!";
std::string ciphertext = encrypt(pubKey, plaintext);
std::cout << "Encrypted message: " << ciphertext << "\n";
// 示例解密过程
std::string decryptedMessage = decrypt(privKey, ciphertext);
std::cout << "Decrypted message: " << decryptedMessage << "\n";
return 0;
}
```
**
阅读全文
相关推荐
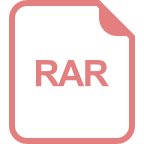
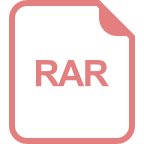



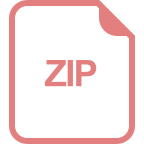
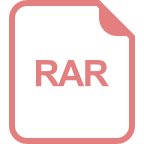
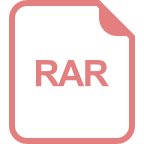
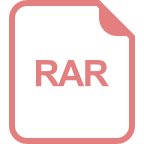
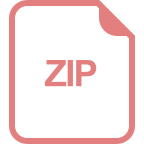
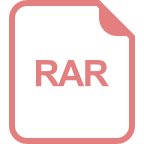
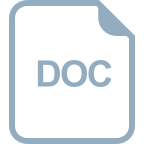
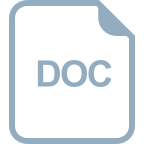
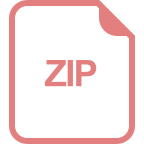
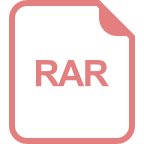
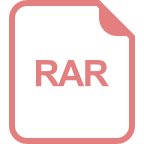
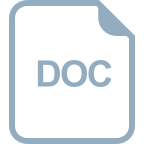