如何使用python制作实时读取串口数据GUI
时间: 2023-09-03 12:10:35 浏览: 291
您可以使用Python中的PySerial库来读取串口数据,并使用Tkinter库创建GUI界面。以下是示例代码:
```python
import serial
import tkinter as tk
# 创建串口对象
ser = serial.Serial('COM1', 9600)
# 创建GUI界面
root = tk.Tk()
# 创建标签用于显示接收到的数据
label = tk.Label(root, text="Waiting for data...")
label.pack()
# 定义函数用于实时读取串口数据并更新标签
def update_label():
# 读取串口数据
data = ser.readline().decode().strip()
# 更新标签显示内容
label.config(text=data)
# 重新调度函数,实现实时更新
root.after(100, update_label)
# 调用函数开始实时更新标签
root.after(100, update_label)
# 运行GUI界面
root.mainloop()
```
该代码创建了一个实时读取串口数据并显示在GUI界面上的程序。其中,串口连接的端口号和波特率可以根据实际情况进行修改。
相关问题
python wxwidgets 串口通信GUI 完整代码
Python 中使用 `wxWidgets` 创建一个串口通信的 GUI,需要结合 `pySerial` 库来实现串口交互。下面是一个简单的示例代码,展示了一个窗口,用户可以选择串口号和波特率,然后可以发送和接收串口数据:
```python
import wx
from pyserial import Serial
class MyFrame(wx.Frame):
def __init__(self, parent=None):
super().__init__(parent, title="Python Serial GUI", size=(400, 300))
self.serial = None
# 初始化串口选择面板
serial_panel = wx.Panel(self)
serial_box_sizer = wx.BoxSizer(wx.VERTICAL)
self.combo_port = wx.ComboBox(serial_panel, choices=Serial().list_ports(), style=wx.CB_READONLY)
self.text_ctrl_baudrate = wx.TextCtrl(serial_panel, value="9600")
send_button = wx.Button(serial_panel, label="Send")
receive_button = wx.Button(serial_panel, label="Receive")
serial_box_sizer.Add(self.combo_port, proportion=1, flag=wx.EXPAND)
serial_box_sizer.Add(wx.StaticText(serial_panel, label="Baud Rate"), flag=wx.LEFT, border=5)
serial_box_sizer.Add(self.text_ctrl_baudrate, flag=wx.EXPAND)
serial_box_sizer.Add(send_button, flag=wx.ALL, border=5)
serial_box_sizer.Add(receive_button, flag=wx.ALL, border=5)
serial_panel.SetSizer(serial_box_sizer)
# 发送按钮事件处理器
def on_send_click(event):
baud_rate = int(self.text_ctrl_baudrate.GetValue())
try:
self.connect_serial(baud_rate)
self.send_data("Hello from GUI!")
except Exception as e:
wx.MessageBox(str(e), "Error", wx.OK | wx.ICON_ERROR)
send_button.Bind(wx.EVT_BUTTON, on_send_click)
# 接收数据处理
def on_receive_click(event):
try:
data = self.receive_data()
wx.MessageBox(f"Received data: {data}", "Message", wx.OK)
except Exception as e:
wx.MessageBox(str(e), "Error", wx.OK | wx.ICON_ERROR)
receive_button.Bind(wx.EVT_BUTTON, on_receive_click)
def connect_serial(self, baud_rate):
self.serial = Serial(port=self.combo_port.GetStringSelection(), baudrate=baud_rate)
self.serial.timeout = 1 # 设置超时时间,防止阻塞
def send_data(self, data):
if self.serial:
self.serial.write(data.encode('utf-8'))
print(f"Sent: {data}")
def receive_data(self):
if self.serial:
response = self.serial.read_until(b'\r\n') # 假设数据是以换行符分隔
return response.decode().strip()
if __name__ == "__main__":
app = wx.App()
frame = MyFrame()
frame.Show(True)
app.MainLoop()
```
这个例子中,我们创建了两个按钮,当点击“Send”按钮时会连接到选定的串口并发送数据,点击“Receive”按钮则尝试从串口读取数据。注意实际使用时需要对异常情况进行处理。
python tkinter 串口实时接收数据
Python Tkinter是Python中的一个GUI库,可以实现图形界面。串口是一种常见的数据传输方式,通过串口接收数据可以实时获取外部设备传来的数据。
Python提供了Pyserial库,可以实现串口通信。在Python Tkinter中使用串口通信可以通过以下步骤实现:
1. 导入Pyserial库,打开串口并设置参数,如波特率、数据位、停止位等。
2. 使用Tkinter的Text控件展示接收到的数据。可以设置Text的insert方法实现实时更新数据。
3. 在程序中使用Thread或Timer等方式,不断地读取串口发送的数据。通过串口的read方法读取数据,将数据写入到Text控件中。
4. 关闭串口和程序,需要及时释放串口资源,避免出现串口被占用等问题。
通过以上步骤,可以在Python Tkinter中实现串口实时接收数据的功能,便于设备数据的监测。
阅读全文
相关推荐
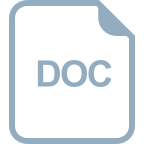
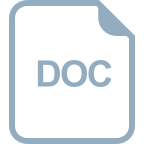
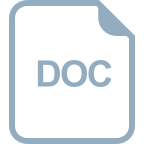
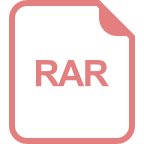
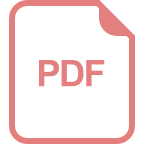
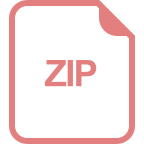
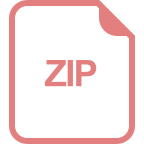
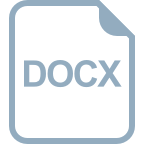
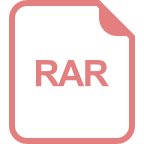







