以二叉链表作为存储结构,建立一棵二叉树; 分别按先序、中序、后序和层次顺序遍历二叉树,并给出代码
时间: 2024-02-04 20:02:03 浏览: 107
好的,以下是使用 C++ 语言实现二叉树的建立和四种遍历方式的代码:
```cpp
#include <iostream>
#include <queue>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 先序遍历,根左右
void preorder(TreeNode* root) {
if (root == NULL) return;
cout << root->val << " ";
preorder(root->left);
preorder(root->right);
}
// 中序遍历,左根右
void inorder(TreeNode* root) {
if (root == NULL) return;
inorder(root->left);
cout << root->val << " ";
inorder(root->right);
}
// 后序遍历,左右根
void postorder(TreeNode* root) {
if (root == NULL) return;
postorder(root->left);
postorder(root->right);
cout << root->val << " ";
}
// 层次遍历
void levelorder(TreeNode* root) {
if (root == NULL) return;
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
TreeNode* cur = q.front();
q.pop();
cout << cur->val << " ";
if (cur->left) q.push(cur->left);
if (cur->right) q.push(cur->right);
}
}
// 建立二叉树
TreeNode* buildTree() {
int val;
cin >> val;
if (val == -1) return NULL;
TreeNode* root = new TreeNode(val);
root->left = buildTree();
root->right = buildTree();
return root;
}
int main() {
cout << "请输入二叉树的节点值,-1表示空节点:" << endl;
TreeNode* root = buildTree();
cout << "先序遍历结果:";
preorder(root);
cout << endl;
cout << "中序遍历结果:";
inorder(root);
cout << endl;
cout << "后序遍历结果:";
postorder(root);
cout << endl;
cout << "层次遍历结果:";
levelorder(root);
cout << endl;
return 0;
}
```
注意:这里输入的二叉树按照先序遍历的方式输入,-1 表示空节点。可以根据需要进行修改。
阅读全文
相关推荐
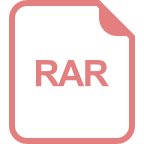














