写一段爬取api接口的代码
时间: 2023-02-22 18:01:32 浏览: 43
这是一段使用 Python 语言爬取 API 接口的代码示例:
```
import requests
url = "https://api.example.com/data"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Failed to retrieve data. Status code: ", response.status_code)
```
这段代码使用 `requests` 库发送一个 GET 请求到 API 接口的 URL,然后判断响应的状态码是否为 200,如果是,就将响应的 JSON 数据解析出来并打印出来;否则,打印错误信息。
相关问题
写一段代码可以爬取百度的图片
以下是一个简单的Python代码,可以爬取百度图片搜索结果的图片:
```python
import requests
import os
# 定义搜索关键词和图片数量
search_word = '猫'
num_images = 10
# 设置请求头部信息
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 创建保存图片的文件夹
if not os.path.exists(search_word):
os.makedirs(search_word)
# 循环获取图片
url = 'http://image.baidu.com/search/acjson'
params = {
'tn': 'resultjson_com',
'ipn': 'rj',
'ct': 201326592,
'fp': 'result',
'queryWord': search_word,
'cl': 2,
'lm': -1,
'ie': 'utf-8',
'oe': 'utf-8',
'adpicid': '',
'st': -1,
'z': '',
'ic': 0,
'word': search_word,
's': '',
'se': '',
'tab': '',
'width': '',
'height': '',
'face': 0,
'istype': 2,
'qc': '',
'nc': 1,
'fr': '',
'pn': 0,
'rn': 30,
'gsm': '1e'}
for i in range(num_images):
params['pn'] = i * 30
response = requests.get(url, headers=headers, params=params)
response_json = response.json()
for image_info in response_json['data']:
if 'thumbURL' in image_info:
image_url = image_info['thumbURL']
response_image = requests.get(image_url, headers=headers)
with open(os.path.join(search_word, '{}.jpg'.format(i * 30 + response_json['data'].index(image_info))),
'wb') as f:
f.write(response_image.content)
```
注意:爬取百度图片有一定的风险,可能会触发反爬虫机制。建议使用合法的方式获取图片,例如使用百度开放平台提供的API接口。
写一段代码爬取王者荣耀的皮肤大图
由于王者荣耀的图片都是在服务器上,所以无法直接通过爬虫获取,但可以通过模拟玩家客户端请求获取到皮肤大图。以下是一个示例代码:
```python
import requests
import json
# 获取皮肤信息接口
skin_api_url = "https://game.gtimg.cn/images/lol/act/img/js/heroList/hero_list.js"
# 请求皮肤信息接口
skin_api_response = requests.get(skin_api_url)
# 解析响应结果
skin_data = json.loads(skin_api_response.text)
# 循环遍历每个英雄
for hero in skin_data['hero']:
# 获取英雄皮肤信息接口
hero_skin_url = f"https://game.gtimg.cn/images/lol/act/img/skin/big{hero['heroId']}{}.jpg"
# 遍历英雄的每个皮肤
for skin in hero['skins']:
# 构造皮肤大图链接
skin_image_url = f"{hero_skin_url[:-5]}{skin['id']}.jpg"
# 请求皮肤大图链接并保存图片
skin_image_response = requests.get(skin_image_url)
with open(f"{hero['name']}-{skin['name']}.jpg", "wb") as f:
f.write(skin_image_response.content)
```
注:由于王者荣耀的图片都是在服务器上,所以此代码仅供学习参考,不可商用。
相关推荐
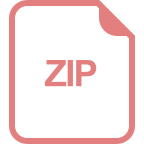
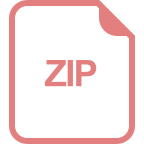












