一个文件夹下有文件也有子文件夹,要求压缩并且原来的层级不变
时间: 2024-04-24 22:24:08 浏览: 207
如果要将文件夹及其子文件夹中的所有文件压缩到一个zip文件中,并保持原有的文件层级结构,可以使用以下代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class FolderCompressor {
private static final int THREAD_POOL_SIZE = 5;
public static void main(String[] args) {
String sourceFolder = "/path/to/source/folder";
String zipFile = "/path/to/output/zip/file.zip";
compressFolder(sourceFolder, zipFile);
}
public static void compressFolder(String sourceFolder, String zipFile) {
File folderToCompress = new File(sourceFolder);
File zipOutputFile = new File(zipFile);
if (!folderToCompress.exists()) {
System.out.println("Source folder does not exist.");
return;
}
try (ZipOutputStream zipOutputStream = new ZipOutputStream(new FileOutputStream(zipOutputFile))) {
ExecutorService executorService = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
compressFolder(folderToCompress, "", zipOutputStream, executorService);
executorService.shutdown();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void compressFolder(File file, String parentPath, ZipOutputStream zipOutputStream, ExecutorService executorService)
throws IOException {
if (file.isDirectory()) {
String folderPath = parentPath + file.getName() + "/";
ZipEntry zipEntry = new ZipEntry(folderPath);
zipOutputStream.putNextEntry(zipEntry);
zipOutputStream.closeEntry();
for (File subFile : file.listFiles()) {
compressFolder(subFile, folderPath, zipOutputStream, executorService);
}
} else {
executorService.execute(() -> {
try (FileInputStream fileInputStream = new FileInputStream(file)) {
ZipEntry zipEntry = new ZipEntry(parentPath + file.getName());
zipOutputStream.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int length;
while ((length = fileInputStream.read(buffer)) > 0) {
zipOutputStream.write(buffer, 0, length);
}
zipOutputStream.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
});
}
}
}
```
请将`/path/to/source/folder`替换为要压缩的文件夹路径,并将`/path/to/output/zip/file.zip`替换为输出的压缩文件路径。该代码会递归遍历文件夹及其子文件夹,并将每个文件和子文件夹分别添加到zip文件中,保持原有的层级结构。使用线程池来并行处理文件和文件夹的压缩操作。
阅读全文
相关推荐
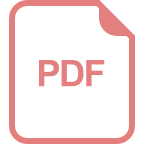
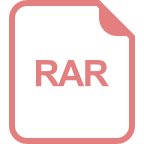
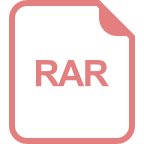
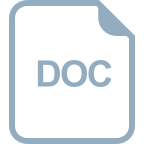
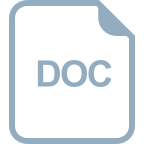
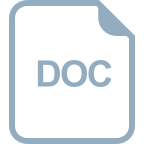
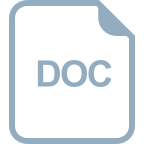
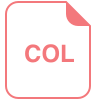
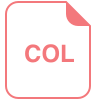
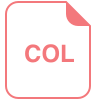
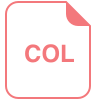
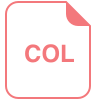
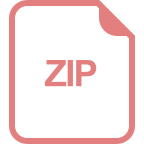
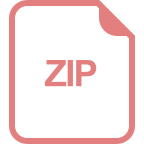
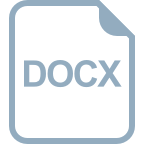
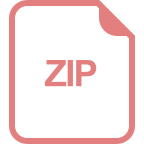