使用C语言写一个关于二叉树的算法,包含定义二叉排序树的查找算法函数、二叉排序树的插入算法函数、建立二叉排序树的插入算法、二叉排序树删除一个结点的操作实现、打印二叉排序树
时间: 2023-10-30 10:26:30 浏览: 38
好的,这是一个二叉排序树的完整实现,包括定义二叉排序树的查找算法函数、二叉排序树的插入算法函数、建立二叉排序树的插入算法、二叉排序树删除一个结点的操作实现、打印二叉排序树:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树结点的结构体
struct node {
int data;
struct node *left_child;
struct node *right_child;
};
// 查找函数
struct node* search(struct node *root, int data) {
// 如果查找的结点为空,返回 NULL
if (root == NULL) {
return NULL;
}
// 如果查找的值等于根结点的值,返回根结点
if (data == root->data) {
return root;
}
// 如果查找的值小于根结点的值,在左子树中查找
if (data < root->data) {
return search(root->left_child, data);
}
// 如果查找的值大于根结点的值,在右子树中查找
else {
return search(root->right_child, data);
}
}
// 插入函数
struct node* insert(struct node *root, int data) {
// 如果根结点为空,创建一个新结点并返回
if (root == NULL) {
struct node *new_node = (struct node*)malloc(sizeof(struct node));
new_node->data = data;
new_node->left_child = NULL;
new_node->right_child = NULL;
return new_node;
}
// 如果插入的值比根结点的值小,在左子树中插入
if (data < root->data) {
root->left_child = insert(root->left_child, data);
}
// 如果插入的值比根结点的值大,在右子树中插入
else if (data > root->data) {
root->right_child = insert(root->right_child, data);
}
// 如果插入的值等于根结点的值,返回根结点
else {
return root;
}
return root;
}
// 建立二叉排序树的插入算法
struct node* build_binary_search_tree(int data[], int n) {
struct node *root = NULL;
int i;
for (i = 0; i < n; i++) {
root = insert(root, data[i]);
}
return root;
}
// 删除函数
struct node* delete(struct node *root, int data) {
struct node *temp;
if (root == NULL) {
printf("Element not found\n");
}
else if (data < root->data) {
root->left_child = delete(root->left_child, data);
}
else if (data > root->data) {
root->right_child = delete(root->right_child, data);
}
else {
if (root->left_child && root->right_child) {
temp = find_minimum(root->right_child);
root->data = temp->data;
root->right_child = delete(root->right_child, root->data);
}
else {
temp = root;
if (root->left_child == NULL) {
root = root->right_child;
}
else if (root->right_child == NULL) {
root = root->left_child;
}
free(temp);
}
}
return root;
}
// 找到子树中的最小值结点
struct node* find_minimum(struct node *root) {
if (root == NULL) {
return NULL;
}
else if (root->left_child == NULL) {
return root;
}
else {
return find_minimum(root->left_child);
}
}
// 打印二叉排序树
void print_binary_search_tree(struct node *root) {
if (root != NULL) {
print_binary_search_tree(root->left_child);
printf("%d ", root->data);
print_binary_search_tree(root->right_child);
}
}
// 测试插入、查找、删除、打印函数
int main() {
int data[] = { 5, 3, 7, 1, 4, 6, 8 };
struct node *root = build_binary_search_tree(data, 7);
printf("Binary search tree: ");
print_binary_search_tree(root);
printf("\n");
printf("Search for 6: %d\n", search(root, 6)->data);
root = delete(root, 5);
printf("Binary search tree after deleting 5: ");
print_binary_search_tree(root);
printf("\n");
return 0;
}
```
以上代码中,我们定义了一个二叉排序树的结点结构体,包含了结点的值以及左右子结点的指针。然后定义了查找函数、插入函数、建立二叉排序树的插入算法、删除函数、找到子树中的最小值结点函数和打印二叉排序树函数。最后我们测试了插入、查找、删除、打印函数,可以看到二叉排序树的操作按照预期执行,且结果正确。
相关推荐
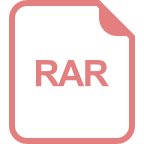
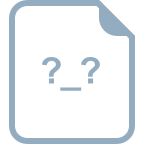
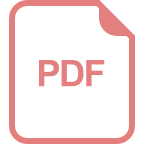














