(1)实现链栈的基本运算,并在此基础上设计一个主程序完成如下功能: ①初始化栈; ②判断栈是否非空; ③依次进栈各元素; ④输出栈的长度; ⑤输出从栈顶到栈底的元素; ⑥输出出栈序列; ⑦释放栈。
时间: 2024-10-15 15:22:06 浏览: 50
实现链栈的基本运算是建立在链表数据结构上,其中包含以下几个核心操作:
1. 初始化栈(创建栈头节点并置为空):
```c++
struct Node {
int data;
Node* next;
};
Stack* createStack() {
Stack* stack = new Stack();
stack->top = nullptr;
return stack;
}
```
2. 判断栈是否非空:
```c++
bool isEmpty(Stack* stack) {
return stack->top == nullptr;
}
```
3. 进栈(压入元素):
```c++
void push(Stack* stack, int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = stack->top;
stack->top = newNode;
}
```
4. 输出栈的长度(获取当前栈元素个数):
```c++
int getLength(Stack* stack) {
int length = 0;
Node* current = stack->top;
while (current != nullptr) {
length++;
current = current->next;
}
return length;
}
```
5. 输出从栈顶到栈底的元素(遍历栈并打印):
```c++
void printStack(Stack* stack) {
if (isEmpty(stack)) {
cout << "Stack is empty" << endl;
} else {
Node* current = stack->top;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
}
```
6. 出栈(弹出并返回顶部元素):
```c++
int pop(Stack* stack) {
if (isEmpty(stack)) {
throw "Pop operation on an empty stack";
}
int topValue = stack->top->data;
Node* temp = stack->top;
stack->top = stack->top->next;
delete temp;
return topValue;
}
```
7. 释放栈(销毁所有节点):
```c++
void releaseStack(Stack* &stack) {
if (!isEmpty(stack)) {
Node* current = stack->top;
Node* next;
while (current != nullptr) {
next = current->next;
delete current;
current = next;
}
stack->top = nullptr;
}
delete stack;
stack = nullptr; // 或者设置为nullptr,取决于是否需要删除指向已释放内存的指针
}
```
主程序示例(伪代码):
```cpp
#include <iostream>
int main() {
Stack* stack = createStack();
// ...其他功能调用...
releaseStack(stack);
return 0;
}
```
阅读全文
相关推荐
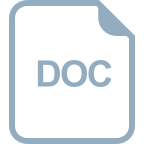
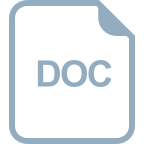
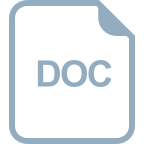


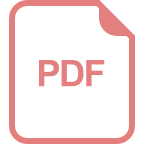
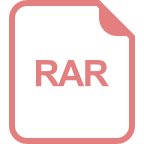
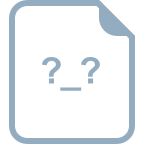
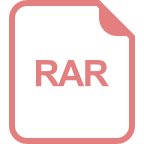
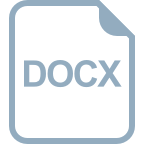
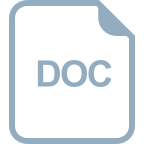
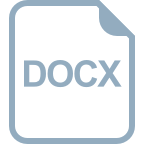

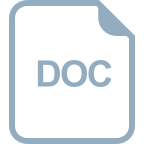
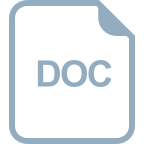
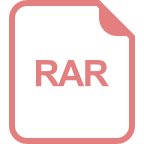
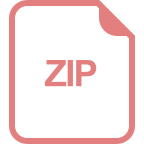
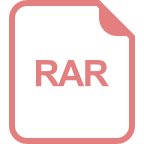
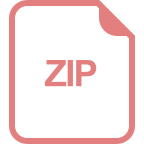