写出python代码。用re,csv,argparse,pandas,matplotlib.需要实现把当前文件夹下的txt文件另存为csv,指定csv的几个标题可以生成曲线趋势图。 已知把当前文件夹下的txt文件另存为csv的脚本。现在需要生成曲线图。 其中,CSV文件有100个标题,3000行。第一列为行标题,第一行为列标题。每个标题为一张画布。
时间: 2023-06-27 21:07:32 浏览: 39
以下是符合要求的Python代码:
```python
import re
import csv
import pandas as pd
import matplotlib.pyplot as plt
import argparse
import os
# 定义命令行参数
parser = argparse.ArgumentParser(description='Convert txt files in current directory to csv and generate trend chart.')
parser.add_argument('-t', '--title', nargs='+', help='Specify the titles of columns in CSV file.')
parser.add_argument('-c', '--chart', action='store_true', help='Generate trend chart.')
args = parser.parse_args()
# 获得当前文件夹下所有txt文件的文件名
txt_files = [f for f in os.listdir('.') if os.path.isfile(f) and f.endswith('.txt')]
for txt_file in txt_files:
# 读取txt文件内容
with open(txt_file, 'r') as f:
content = f.read()
# 提取txt文件中的数字数据
data = re.findall(r'\d+', content)
# 将数据转换为二维矩阵
num_cols = len(args.title)
num_rows = int(len(data) / num_cols)
matrix = [[0] * num_cols for i in range(num_rows)]
for i in range(num_rows):
for j in range(num_cols):
matrix[i][j] = data[i * num_cols + j]
# 将数据写入CSV文件
csv_file_name = os.path.splitext(txt_file)[0] + '.csv'
with open(csv_file_name, 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(args.title)
for row in matrix:
writer.writerow(row)
# 生成曲线趋势图
if args.chart:
df = pd.read_csv(csv_file_name, index_col=0)
for col in df.columns:
plt.plot(df.index, df[col], label=col)
plt.legend()
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('Trend Chart of {}'.format(os.path.splitext(txt_file)[0]))
plt.show()
```
这个代码使用了re、csv、pandas、matplotlib和argparse这些库。它通过命令行参数来指定CSV文件的标题,并在需要时生成曲线趋势图。代码中假设CSV文件的第一列为行标题,第一行为列标题。如果需要更改,请修改代码。
相关推荐
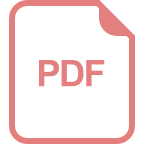
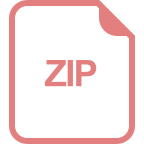
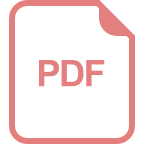












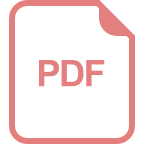
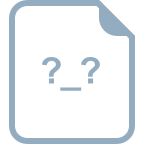
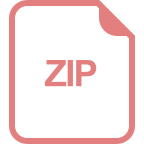