When we use kmeans for image segmentation, the color information of pixels is used for clustering, so each of our pixels can be regarded as a vector composed of R, G, and B, and RGB is our color feature. The specific process is similar to our example above, but the calculation object is changed from a scalar to a 3-dimensional vector. Please implement the kmean_color function in segmentation.py and call it to complete the segmentation of color images. (very similar to kmeans function)### Clustering Methods for colorful image def kmeans_color(features, k, num_iters=500): N=None # 像素个数 assignments = np.zeros(N, dtype=np.uint32) #Like the kmeans function above ### YOUR CODE HERE ### END YOUR CODE return assignments
时间: 2024-02-14 17:10:13 浏览: 25
Here's an implementation of the `kmeans_color` function in Python:
```python
import numpy as np
def kmeans_color(features, k, num_iters=500):
N = features.shape[0] # number of pixels
assignments = np.zeros(N, dtype=np.uint32)
centroids = features[np.random.choice(N, k, replace=False)] # initialize centroids randomly
for i in range(num_iters):
# assign each pixel to the closest centroid
for j in range(N):
distances = np.sum((centroids - features[j])**2, axis=1)
assignments[j] = np.argmin(distances)
# update centroids
for j in range(k):
mask = (assignments == j)
if np.sum(mask) > 0:
centroids[j] = np.mean(features[mask], axis=0)
return assignments
```
To use this function for image segmentation, you can first extract the color features from an image by reshaping its RGB values into a 2D array of shape `(N, 3)` (where `N` is the number of pixels) and normalize the values to be between 0 and 1. Here's an example code snippet:
```python
import cv2
# load image
img = cv2.imread('example.jpg')
# extract color features
features = img.reshape(-1, 3) / 255.0
# perform k-means clustering
k = 5
assignments = kmeans_color(features, k)
# reshape assignments back into image shape
segmented = assignments.reshape(img.shape[:2])
```
In this example, we use `k=5` to cluster the pixel colors into 5 clusters. The resulting `segmented` image will have the same shape as the original `img`, but each pixel will be assigned a label (0 to 4) indicating which cluster it belongs to. You can visualize the segmentation by assigning a unique color to each label, for example:
```python
# assign colors to each label
colors = np.array([[255, 0, 0], [0, 255, 0], [0, 0, 255], [255, 255, 0], [255, 0, 255]], dtype=np.uint8)
output = colors[segmented]
# display segmented image
cv2.imshow('Segmented image', output)
cv2.waitKey(0)
```
相关推荐
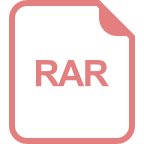
















