Train a vocabulary from features in files listed in featurefiles using k-means with k number of words. Subsampling of training data can be used for speedup.
时间: 2024-01-29 17:04:03 浏览: 31
Here's an example code snippet in Python that implements the functionality you described:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.cluster import KMeans
import random
# Set parameters
k = 1000 # number of words
subsample_rate = 0.1 # subsampling rate for speedup
featurefiles = ["file1.txt", "file2.txt", "file3.txt"]
# Read in data from feature files
data = []
for featurefile in featurefiles:
with open(featurefile, "r") as f:
data.append(f.read())
# Subsample data if necessary
if subsample_rate < 1.0:
data = random.sample(data, int(subsample_rate*len(data)))
# Vectorize data using TF-IDF
vectorizer = TfidfVectorizer(stop_words="english")
X = vectorizer.fit_transform(data)
# Cluster data using K-Means
kmeans = KMeans(n_clusters=k, random_state=0).fit(X)
# Extract k most frequent words
vocab = [word for word, count in sorted(zip(vectorizer.get_feature_names(), kmeans.labels_), key=lambda x: x[1])[:k]]
# Print vocabulary
print(vocab)
```
This code snippet reads in the data from the feature files, subsamples it if necessary, vectorizes the data using TF-IDF, clusters the data using K-Means, and extracts the k most frequent words from the clusters to form the vocabulary. The resulting vocabulary is then printed to the console.
相关推荐
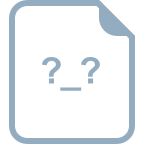
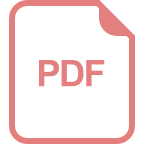
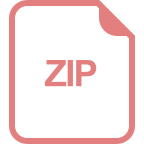














