Implement about, which takes a list of topic words. It returns a function that can be passed to choose as the select argument. The returned function takes a paragraph and returns a boolean indicating whether that paragraph contains any of the words in topic. To make this comparison accurately, you will need to ignore case (that is, assume that uppercase and lowercase letters don't change what word it is) and punctuation. Assume that all words in the topic list are already lowercased and do not contain punctuation.
时间: 2024-02-25 20:56:41 浏览: 21
Sure, here's an implementation in Python:
```python
import re
def about(topic):
# compile a regular expression to match any of the topic words
pattern = re.compile("|".join(re.escape(word) for word in topic), re.IGNORECASE)
def contains_topic(paragraph):
# remove all punctuation from the paragraph
paragraph = re.sub(r"\p{P}+", "", paragraph)
# check if the paragraph contains any of the topic words
return bool(pattern.search(paragraph))
return contains_topic
```
Here's an explanation of how it works:
- `re.compile("|".join(re.escape(word) for word in topic), re.IGNORECASE)` creates a regular expression that matches any of the topic words, ignoring case.
- `def contains_topic(paragraph)` defines a function that takes a paragraph and checks if it contains any of the topic words.
- `re.sub(r"\p{P}+", "", paragraph)` removes all punctuation from the paragraph using a regular expression.
- `bool(pattern.search(paragraph))` checks if any of the topic words match the paragraph, returning `True` if there is a match and `False` otherwise.
- `return contains_topic` returns the function that checks for topic words in a paragraph.
To use it, you could create a list of topic words and pass it to `about` to create a function that checks for those words:
```python
topic = ["python", "programming", "code"]
contains_topic = about(topic)
paragraph = "I love programming in Python. Writing code is so much fun!"
if contains_topic(paragraph):
print("This paragraph is about programming!")
else:
print("This paragraph is not about programming.")
```
Output:
```
This paragraph is about programming!
```
相关推荐















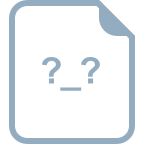
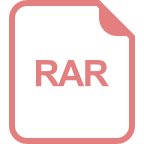
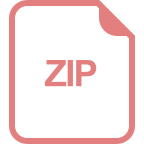
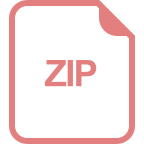