用python实现五路视觉传感器循迹小车,写出其代码
时间: 2024-11-30 11:19:41 浏览: 9
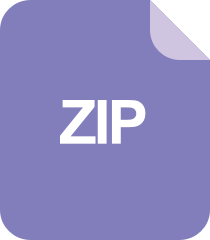
VREP与python实现基于视觉的小车PID循迹
在Python中实现一个基于五路视觉传感器的小车循迹系统通常涉及到摄像头采集图像、图像处理(比如边缘检测或颜色识别)、以及控制车辆移动的部分。这里提供一个简化版的基本框架,实际应用可能需要结合硬件库如picamera、OpenCV等:
```python
import cv2
import numpy as np
from time import sleep
# 定义车辆移动速度
VELOCITY = 50
# 设定五个光敏电阻的阈值范围
THRESHOLD_VALUES = [20, 40, 60, 80, 100]
class VisionSensorCar:
def __init__(self):
self.camera = cv2.VideoCapture(0)
self.width = int(self.camera.get(cv2.CAP_PROP_FRAME_WIDTH))
self.height = int(self.camera.get(cv2.CAP_PROP_FRAME_HEIGHT))
def process_image(self, image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, THRESHOLD_VALUES[0], THRESHOLD_VALUES[4])
lines = cv2.HoughLinesP(edges, rho=1, theta=np.pi/180, threshold=THRESHOLD_VALUES[1], minLineLength=self.width//10, maxLineGap=self.width//20)
# 根据线的位置判断行驶方向
direction = self.analyze_lines(lines)
return direction
def analyze_lines(self, lines):
if lines is not None and len(lines) > 0:
# 根据线的方向计算移动命令
pass
else:
return "center" # 当无明确指引时,假设向中心行驶
def move_car(self, direction):
if direction == "left":
print("Moving left")
elif direction == "right":
print("Moving right")
else:
print("Moving straight")
def run(self):
while True:
ret, frame = self.camera.read()
if ret:
direction = self.process_image(frame)
self.move_car(direction)
sleep(VELOCITY / 1000) # 控制帧率
else:
break
self.camera.release()
if __name__ == "__main__":
car = VisionSensorCar()
car.run()
```
这个代码只是一个基本的框架,实际实现可能会更复杂,包括线段跟踪算法、路径决策机制等。你需要根据实际使用的摄像头型号、光照条件和传感器特性调整参数,并可能还需要连接电机或其他驱动设备来控制小车的实际运动。
阅读全文
相关推荐
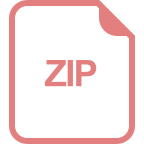



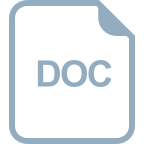
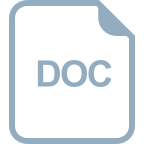



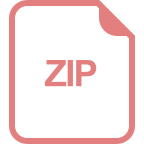
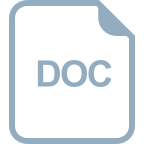
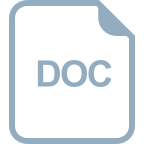



