告诉我上述回答的 表的脚本 然后pom 然后java代码
时间: 2024-02-23 15:57:54 浏览: 101
好的,我可以给您提供一份示例代码,供您参考:
1. 数据库表的脚本
这里我们可以使用MySQL数据库,创建一个名为file_info的表,用于存储文件的相关信息。表结构如下:
```
CREATE TABLE `file_info` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`file_name` varchar(255) DEFAULT NULL,
`file_path` varchar(255) DEFAULT NULL,
`file_size` bigint(20) DEFAULT NULL,
`create_time` datetime DEFAULT NULL,
`update_time` datetime DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
2. Maven依赖配置
在pom.xml文件中添加以下依赖:
```
<dependencies>
<!-- Spring Boot Web Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Data JPA Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- MySQL Connector/J -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- Spring Boot Actuator -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
</dependencies>
```
3. Java代码实现
在Java代码中,我们可以创建一个名为FileController的类,用于处理文件相关的API请求。代码如下:
```
@RestController
@RequestMapping("/api/file")
public class FileController {
@Autowired
private FileRepository fileRepository;
// 上传文件
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file) {
try {
// 获取文件名
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
// 创建文件实体对象
FileInfo fileInfo = new FileInfo();
fileInfo.setFileName(fileName);
fileInfo.setFilePath("/uploads/" + fileName);
fileInfo.setFileSize(file.getSize());
fileInfo.setCreateTime(new Date());
fileInfo.setUpdateTime(new Date());
// 保存文件到磁盘
Path path = Paths.get("uploads/" + fileName);
Files.copy(file.getInputStream(), path, StandardCopyOption.REPLACE_EXISTING);
// 保存文件信息到数据库
fileRepository.save(fileInfo);
return ResponseEntity.ok().body("File uploaded successfully!");
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to upload file!");
}
}
// 下载文件
@GetMapping("/download/{id}")
public ResponseEntity<Resource> downloadFile(@PathVariable Integer id, HttpServletRequest request) {
// 获取文件实体对象
Optional<FileInfo> fileInfoOptional = fileRepository.findById(id);
if (!fileInfoOptional.isPresent()) {
return ResponseEntity.notFound().build();
}
FileInfo fileInfo = fileInfoOptional.get();
// 加载文件到内存
Resource resource = null;
try {
Path path = Paths.get("uploads/" + fileInfo.getFileName());
resource = new UrlResource(path.toUri());
} catch (MalformedURLException e) {
e.printStackTrace();
}
// 设置文件下载响应头
String contentType = null;
try {
contentType = request.getServletContext().getMimeType(resource.getFile().getAbsolutePath());
} catch (IOException e) {
e.printStackTrace();
}
if (contentType == null) {
contentType = "application/octet-stream";
}
return ResponseEntity.ok()
.contentType(MediaType.parseMediaType(contentType))
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + fileInfo.getFileName() + "\"")
.body(resource);
}
// 删除文件
@DeleteMapping("/{id}")
public ResponseEntity<?> deleteFile(@PathVariable Integer id) {
// 获取文件实体对象
Optional<FileInfo> fileInfoOptional = fileRepository.findById(id);
if (!fileInfoOptional.isPresent()) {
return ResponseEntity.notFound().build();
}
FileInfo fileInfo = fileInfoOptional.get();
// 删除磁盘文件
Path path = Paths.get("uploads/" + fileInfo.getFileName());
try {
Files.deleteIfExists(path);
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to delete file!");
}
// 删除数据库中的文件信息
fileRepository.deleteById(id);
return ResponseEntity.ok().body("File deleted successfully!");
}
// 获取文件列表
@GetMapping("/")
public ResponseEntity<List<FileInfo>> getFileList(Pageable pageable) {
Page<FileInfo> filePage = fileRepository.findAll(pageable);
List<FileInfo> fileList = filePage.getContent();
return ResponseEntity.ok().body(fileList);
}
}
```
其中,FileRepository是一个JPA接口,用于操作file_info表。代码如下:
```
@Repository
public interface FileRepository extends JpaRepository<FileInfo, Integer> {
}
```
最后,我们还需要创建一个名为FileInfo的实体类,用于映射file_info表的数据。代码如下:
```
@Entity
@Table(name = "file_info")
public class FileInfo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "file_name")
private String fileName;
@Column(name = "file_path")
private String filePath;
@Column(name = "file_size")
private Long fileSize;
@Column(name = "create_time")
private Date createTime;
@Column(name = "update_time")
private Date updateTime;
// 省略getter和setter方法
}
```
以上就是一个简单的局域网文件管理共享的示例代码,供您参考。
阅读全文
相关推荐
















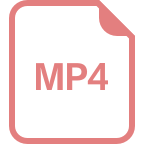