python动态规划法求解tsp代码实例
时间: 2024-11-07 09:11:06 浏览: 0
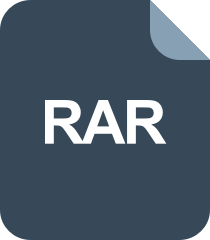
tsp.rar_TSP问题实例_TSP问题的例子_tsp
旅行商问题(Traveling Salesman Problem, TSP)是一个经典的组合优化问题,Python中的动态规划解决方案通常涉及使用贪心策略结合回溯法来近似解决。下面是一个简单的例子,使用了Kruskal's 算法作为启发式方法,并不是完全的动态规划,因为TSP本身是一个NP-hard问题,无法保证全局最优解。
```python
import heapq
# 假设我们有这些城市及其之间的距离矩阵
cities = [('A', 'B', 7), ('A', 'C', 9), ('B', 'C', 14), ('B', 'D', 10),
('C', 'D', 18), ('D', 'E', 2), ('C', 'F', 4), ('D', 'F', 7),
('E', 'F', 9)]
def tsp(cities):
# 使用 Kruskal's 算法构建初始路径
edges = [(cost, (u, v)) for u, _, cost in cities]
graph = dict(sorted(edges))
mst_cost = 0
mst_edges = []
while len(mst_edges) < len(cities)-1:
edge = min((graph.pop((u, v)), u, v) for u, v in graph if u != v)
mst_cost += edge[0]
mst_edges.append(edge[1:])
# 将起始点添加到路径并计算成本
path = [mst_edges[0][0]]
current_city = path[-1]
total_cost = mst_cost
# 添加其他城市的回溯搜索
unvisited_cities = set(city[0] for city in cities)
while unvisited_cities:
next_city = min(unvisited_cities, key=lambda c: cities[(current_city, c)][1])
path.append(next_city)
total_cost += cities[(current_city, next_city)][2]
current_city = next_city
unvisited_cities.remove(current_city)
return total_cost, path
result = tsp(cities)
print("Total cost:", result[0])
print("Route:", result[1])
```
这个例子仅作为演示,实际应用中可能需要使用更复杂的搜索算法(如分支定界法)来改善结果。注意,这并不是最优解算法,因为Kruskal's算法得到的是一个近似解,而不是真实的TSP解。真正的TSP问题没有现成的简捷算法能在多项式时间内找到全球最优解。
阅读全文
相关推荐
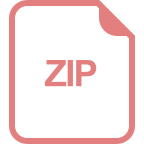
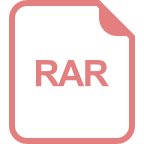















