c语言自动售货机代码
时间: 2024-06-13 21:01:38 浏览: 26
C语言自动售货机代码的实现需要涉及到以下几个方面:
1. 用户界面设计:需要设计一个良好的用户交互界面,包括菜单、输入和输出等。
2. 数据存储和处理:需要将商品信息、库存数量、价格等数据进行存储和处理,可以使用数组、结构体等数据类型。
3. 支付系统设计:需要实现用户支付功能,包括读取用户输入的金额、计算找零、更新库存等。
以下是一个简单的C语言自动售货机代码示例,仅供参考:
```
#include <stdio.h>
#define MAX_ITEMS 10 // 最大库存数量
typedef struct {
char name[20]; // 商品名称
int price; // 商品价格
int stock; // 商品库存数量
} item;
item items[MAX_ITEMS] = { // 商品信息列表
{"Coke", 10, 5},
{"Pepsi", 8, 7},
{"Sprite", 9, 3},
{"Water", 5, 10},
{"Juice", 12, 2}
};
int main() {
int choice, money, total = 0;
printf("Welcome to the vending machine!\n");
while (1) {
// 显示菜单
printf("Menu:\n");
for (int i = 0; i < MAX_ITEMS; i++) {
printf("%d. %s - $%d\n", i + 1, items[i].name, items[i].price);
}
// 输入选择
printf("Enter your choice (0 to exit): ");
scanf("%d", &choice);
if (choice == 0) {
break;
}
if (choice < 1 || choice > MAX_ITEMS) {
printf("Invalid choice!\n");
continue;
}
// 检查库存
if (items[choice - 1].stock == 0) {
printf("Sorry, this item is out of stock!\n");
continue;
}
// 输入金额
printf("Enter your money: ");
scanf("%d", &money);
// 检查金额是否足够
if (money < items[choice - 1].price) {
printf("Not enough money!\n");
continue;
}
// 计算找零
int change = money - items[choice - 1].price;
printf("Change: $%d\n", change);
total += items[choice - 1].price;
items[choice - 1].stock--;
}
printf("Total sales: $%d\n", total);
return 0;
}
```
相关推荐
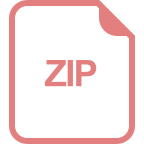










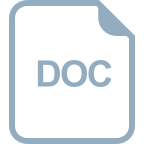
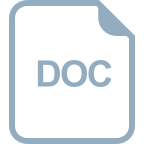