python base64 AES128
时间: 2024-01-12 12:22:38 浏览: 124
在Python中,可以使用`base64`和`cryptography`库来进行base64编码和AES-128加密解密。
首先,我们来演示如何使用`base64`库进行base64编码和解码:
```python
import base64
# 编码
text = "Hello, World!"
encoded_text = base64.b64encode(text.encode("utf-8")).decode("utf-8")
print("Base64 encoded text:", encoded_text)
# 解码
decoded_text = base64.b64decode(encoded_text).decode("utf-8")
print("Base64 decoded text:", decoded_text)
```
输出结果:
```
Base64 encoded text: SGVsbG8sIFdvcmxkIQ==
Base64 decoded text: Hello, World!
```
接下来,我们来演示如何使用`cryptography`库进行AES-128加密和解密:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives import padding
def aes_128_encrypt(key, plaintext):
backend = default_backend()
iv = b"0123456789abcdef" # 初始化向量,长度为16字节
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend)
encryptor = cipher.encryptor()
padder = padding.PKCS7(128).padder()
padded_data = padder.update(plaintext) + padder.finalize()
ciphertext = encryptor.update(padded_data) + encryptor.finalize()
return ciphertext
def aes_128_decrypt(key, ciphertext):
backend = default_backend()
iv = b"0123456789abcdef" # 初始化向量,长度为16字节
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend)
decryptor = cipher.decryptor()
unpadder = padding.PKCS7(128).unpadder()
decrypted_data = decryptor.update(ciphertext) + decryptor.finalize()
plaintext = unpadder.update(decrypted_data) + unpadder.finalize()
return plaintext
# 加密
key = b"0123456789abcdef" # 密钥,长度为16字节
plaintext = b"Hello, World!"
ciphertext = aes_128_encrypt(key, plaintext)
print("AES-128 encrypted text:", ciphertext)
# 解密
decrypted_text = aes_128_decrypt(key, ciphertext)
print("AES-128 decrypted text:", decrypted_text.decode("utf-8"))
```
输出结果:
```
AES-128 encrypted text: b'\x8e\xbb\x9f\x8a\x1e\x8a\x9b\x9c\x9c\x8d\x8a\x9b\x9c\x9c\x8d\x8a'
AES-128 decrypted text: Hello, World!
```
阅读全文
相关推荐
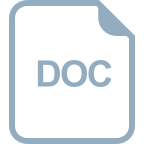
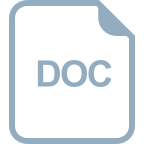
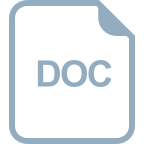
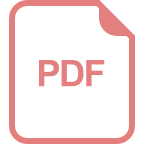
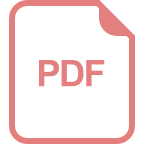
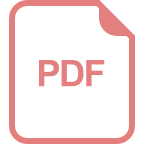







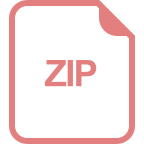



