Description (线性表)逆序建立一个链表,带头结点 Input 输入链表长度n:5 输入数据:1234 5 Output 54321 Sample Input 6 1029788 Sample Output 8879210c语言
时间: 2024-09-06 10:01:05 浏览: 59
逆序建立链表是一种常见的数据结构操作,其目的是将输入的元素顺序反转存储在链表中。在C语言中实现这一过程,首先需要定义链表的结构体,然后编写相应的函数来完成链表的创建和元素的逆序插入。以下是一个简单的实现示例:
首先定义链表节点结构体和相关操作函数:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域
} Node;
// 创建带头结点的链表
Node* createList(int n) {
Node* head = (Node*)malloc(sizeof(Node)); // 创建头结点
head->next = NULL; // 初始化为空链表
Node* tail = head; // 尾指针初始化为头结点,方便后续操作
for (int i = 0; i < n; ++i) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
scanf("%d", &newNode->data); // 输入节点数据
newNode->next = NULL; // 新节点的指针域置为空
tail->next = newNode; // 将新节点链接到链表尾部
tail = newNode; // 更新尾指针
}
return head;
}
// 打印链表(逆序输出)
void printList(Node* head) {
Node* p = head->next; // 从第一个实际节点开始
while (p) {
printf("%d", p->data);
p = p->next; // 向后移动
}
printf("\n");
}
// 释放链表内存
void freeList(Node* head) {
Node* p = head;
while (p) {
Node* temp = p;
p = p->next;
free(temp);
}
}
int main() {
int n;
scanf("%d", &n); // 输入链表长度
Node* list = createList(n); // 创建链表
printList(list); // 输出链表(逆序输出)
freeList(list); // 释放链表内存
return 0;
}
```
在上述代码中,`createList` 函数用于创建链表,`printList` 函数用于逆序打印链表中的元素,`freeList` 函数用于释放链表占用的内存资源。在`main`函数中,我们先读取链表长度`n`,然后调用`createList`函数创建链表,并通过`printList`函数逆序输出链表中的元素。
阅读全文
相关推荐
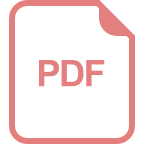
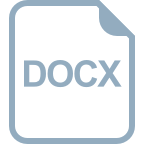
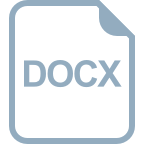

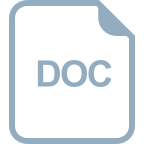
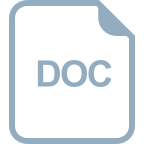
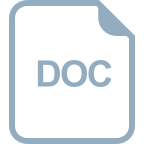
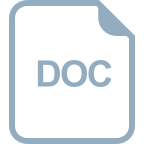










