pytorch如何用cudnn加速
时间: 2023-05-23 13:01:48 浏览: 377
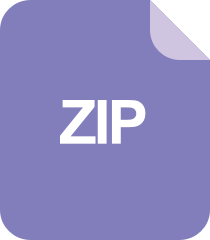
用pytorch实现dnn

PyTorch可以通过使用cuDNN来加速深度学习计算,cuDNN是NVIDIA基于CUDA生态系统的一个加速库,专为DNN(深度神经网络)而设计。在PyTorch中,只需使用nn.backends.cudnn加速器即可使用cuDNN。您可以将以下代码用于加速:
导入torch.nn.backends.cudnn并设置torch.backends.cudnn.benchmark=True,并且您可以确保您的模型在cuda设备上。
import torch
import torch.nn as nn
import torch.nn.functional as F
# Set the device (use GPU if available)
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# Enable the benchmark mode for cuDNN
torch.backends.cudnn.benchmark = True
# Create your model
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(128 * 7 * 7, 512)
self.fc2 = nn.Linear(512, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2)
x = x.view(-1, 128 * 7 * 7)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
model = MyModel().to(device)
# Create your optimizer
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
# Create your loss function
criterion = nn.CrossEntropyLoss()
# Train your model with data
for epoch in range(10):
for i, (inputs, labels) in enumerate(train_loader):
inputs, labels = inputs.to(device), labels.to(device)
outputs = model(inputs)
loss = criterion(outputs, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
阅读全文
相关推荐
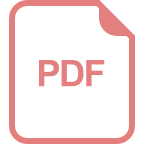



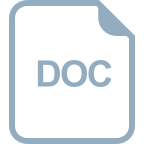


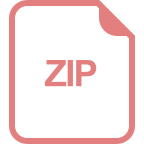
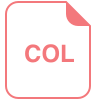





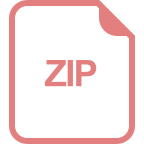

