matlab编写一个函数文件,要求输入x,输出y = cosx + sinx – 2*tanx中的y值。
时间: 2024-09-19 12:13:13 浏览: 67
在MATLAB中,你可以创建一个名为`cos_plus_sin_minus_tan.m`的函数文件来计算给定输入`x`的表达式 `y = cos(x) + sin(x) - 2*tan(x)`。下面是一个简单的函数定义示例:
```matlab
function y = cos_plus_sin_minus_tan(x)
% 定义函数cos_plus_sin_minus_tan
% 输入:x (角度)
% 输出:y (y = cos(x) + sin(x) - 2*tan(x))
% 检查输入是否合理
if ~isnumeric(x) || isnan(x) || x < 0
error('Input x must be a non-negative numeric value.');
end
% 计算y
y = cos(x) + sin(x) - 2 * tan(x);
end
```
使用这个函数的方式如下:
```matlab
x_value = 1; % 可以替换为你想要的任意数值
y_result = cos_plus_sin_minus_tan(x_value);
disp(['The result for y is: ', num2str(y_result)]);
```
相关问题
c语言,输入x后按下式计算y值并输出。 当0≤x≤1时,y=x+cosx 当x<0或x>1时,y=x+sinx
```c
#include <stdio.h>
#include <math.h>
int main() {
double x, y;
printf("Please enter x: ");
scanf("%lf", &x);
if (x >= 0 && x <= 1) {
y = x * cos(x);
} else {
y = x * sin(x);
}
printf("y = %lf\n", y);
return 0;
}
```
)使用matplotlib模块对数学函数进行可视化,所要画出的数学函数有如下6个: y=sinx2+x y=cosx3+2 y=e**(-x)+x**2+1 y=tan|x|+3 y=sinx y=cosx X轴上点的分布范围为,a是取值范围为[-aΠ,aΠ]的随机浮点数,小数点后取两位,该随机数通过交互界面中的按钮1进行实现;每个函数中分布点的个数n为一个整型随机数,该随机数的取值范围为[100,300],该数通过交互界面中的按钮2进行实现。生成图像的要求: 1、六个函数生成多轴域图,按照三行两列的分布形式进行显示; 2、每个函数图的线条颜色都不相同; 3、每个函数图中都应具有该函数的图例,即显示出所对应线条的函数公式; 4、函数图像的生成需通过交互界面中按钮3进行实现。
以下是实现该功能的Python代码,使用了matplotlib模块:
```python
import random
import matplotlib.pyplot as plt
import numpy as np
a = None
n = None
fig, axs = plt.subplots(3, 2, figsize=(10, 12))
def generate_data():
global a, n
a = round(random.uniform(-1, 1) * np.pi, 2)
n = random.randint(100, 300)
def plot_func(func, ax, label):
x = np.linspace(-a*np.pi, a*np.pi, n)
y = func(x)
color = np.random.rand(3,)
ax.plot(x, y, color=color)
ax.legend([label])
def plot_all_funcs():
plot_func(lambda x: np.power(np.sin(x), 2) + x, axs[0, 0], "$y = sin^2(x) + x$")
plot_func(lambda x: np.power(np.cos(x), 3) + 2, axs[0, 1], "$y = cos^3(x) + 2$")
plot_func(lambda x: np.exp(-x) + np.power(x, 2) + 1, axs[1, 0], "$y = e^{-x} + x^2 + 1$")
plot_func(lambda x: np.tan(np.abs(x)) + 3, axs[1, 1], "$y = tan(|x|) + 3$")
plot_func(np.sin, axs[2, 0], "$y = sin(x)$")
plot_func(np.cos, axs[2, 1], "$y = cos(x)$")
plt.show()
generate_data()
btn1 = widgets.Button(description='Generate Data')
btn1.on_click(lambda btn: generate_data())
display(btn1)
btn2 = widgets.Button(description='Plot Functions')
btn2.on_click(lambda btn: plot_all_funcs())
display(btn2)
```
在这个实现中,我们首先定义了一个`generate_data()`函数,用于生成随机的`a`和`n`值,这个函数会在点击“Generate Data”按钮时被调用。
然后,我们定义了一个`plot_func()`函数,用于根据给定的函数绘制图像,并将其添加到指定的子图中。在这个函数中,我们首先根据`a`和`n`生成一组`x`和`y`的值,然后使用`np.random.rand()`函数生成一个随机的RGB颜色,最后使用`ax.plot()`函数绘制函数图像,并将其添加到指定的子图中。
最后,我们定义了一个`plot_all_funcs()`函数,用于绘制所有的函数图像。在这个函数中,我们分别调用`plot_func()`函数绘制六个函数的图像,并将它们添加到指定的子图中。然后,我们使用`plt.show()`函数显示所有的子图。
最后,我们创建了两个按钮,一个用于生成随机数据,另一个用于绘制函数图像。这两个按钮都是使用`ipywidgets`模块创建的,可以在Jupyter Notebook中直接显示和使用。
阅读全文
相关推荐
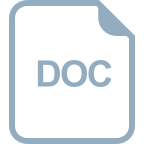
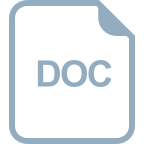
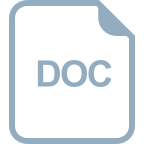













