生命游戏C++easyx
时间: 2023-07-01 18:10:22 浏览: 83
生命游戏(Conway's Game of Life)是一种模拟细胞自动机的经典算法,可以用来模拟生命的繁殖和死亡,是计算机科学中的经典问题之一。这里给出一个使用C++和easyx图形库实现的生命游戏代码。
首先需要安装easyx图形库,在Windows系统下可以在官网下载并安装。
代码如下:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
// 定义棋盘大小
const int BOARD_WIDTH = 800;
const int BOARD_HEIGHT = 600;
const int CELL_SIZE = 10;
// 定义细胞状态
const int DEAD = 0;
const int ALIVE = 1;
// 定义细胞颜色
const COLORREF DEAD_COLOR = RGB(255, 255, 255); // 白色
const COLORREF ALIVE_COLOR = RGB(0, 0, 0); // 黑色
// 定义细胞数组
int cells[BOARD_WIDTH / CELL_SIZE][BOARD_HEIGHT / CELL_SIZE];
// 初始化细胞数组
void initCells() {
for (int i = 0; i < BOARD_WIDTH / CELL_SIZE; i++) {
for (int j = 0; j < BOARD_HEIGHT / CELL_SIZE; j++) {
cells[i][j] = DEAD;
}
}
}
// 绘制棋盘
void drawBoard() {
setbkcolor(WHITE);
cleardevice();
for (int i = 0; i <= BOARD_WIDTH; i += CELL_SIZE) {
line(i, 0, i, BOARD_HEIGHT);
}
for (int j = 0; j <= BOARD_HEIGHT; j += CELL_SIZE) {
line(0, j, BOARD_WIDTH, j);
}
}
// 绘制细胞
void drawCell(int x, int y, int state) {
COLORREF color = state == DEAD ? DEAD_COLOR : ALIVE_COLOR;
setfillcolor(color);
setlinecolor(color);
fillrectangle(x * CELL_SIZE + 1, y * CELL_SIZE + 1, (x + 1) * CELL_SIZE - 1, (y + 1) * CELL_SIZE - 1);
}
// 获取周围8个细胞的状态
int getNeighborState(int x, int y) {
int count = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (i == 0 && j == 0) continue;
int nx = x + i;
int ny = y + j;
if (nx < 0 || ny < 0 || nx >= BOARD_WIDTH / CELL_SIZE || ny >= BOARD_HEIGHT / CELL_SIZE) continue;
if (cells[nx][ny] == ALIVE) count++;
}
}
return count;
}
// 更新细胞状态
void updateCells() {
int newCells[BOARD_WIDTH / CELL_SIZE][BOARD_HEIGHT / CELL_SIZE];
for (int i = 0; i < BOARD_WIDTH / CELL_SIZE; i++) {
for (int j = 0; j < BOARD_HEIGHT / CELL_SIZE; j++) {
int neighborCount = getNeighborState(i, j);
if (cells[i][j] == ALIVE) {
if (neighborCount == 2 || neighborCount == 3) newCells[i][j] = ALIVE;
else newCells[i][j] = DEAD;
}
else {
if (neighborCount == 3) newCells[i][j] = ALIVE;
else newCells[i][j] = DEAD;
}
}
}
for (int i = 0; i < BOARD_WIDTH / CELL_SIZE; i++) {
for (int j = 0; j < BOARD_HEIGHT / CELL_SIZE; j++) {
cells[i][j] = newCells[i][j];
}
}
}
// 随机生成细胞状态
void randomCells() {
srand((unsigned int)time(NULL));
for (int i = 0; i < BOARD_WIDTH / CELL_SIZE; i++) {
for (int j = 0; j < BOARD_HEIGHT / CELL_SIZE; j++) {
int state = rand() % 2;
cells[i][j] = state;
drawCell(i, j, state);
}
}
}
int main()
{
initgraph(BOARD_WIDTH, BOARD_HEIGHT);
initCells();
randomCells();
while (!_kbhit()) {
drawBoard();
updateCells();
for (int i = 0; i < BOARD_WIDTH / CELL_SIZE; i++) {
for (int j = 0; j < BOARD_HEIGHT / CELL_SIZE; j++) {
drawCell(i, j, cells[i][j]);
}
}
Sleep(100);
}
closegraph();
return 0;
}
```
代码中使用了easyx图形库的相关函数来绘制棋盘和细胞。随机生成细胞状态后,不断更新细胞状态并重新绘制即可实现生命游戏的效果。
在代码中可以根据需要调整棋盘大小、细胞大小和更新速度等参数。
阅读全文
相关推荐
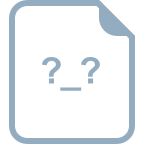
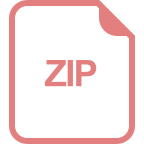
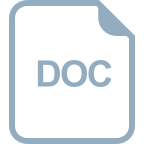



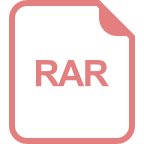
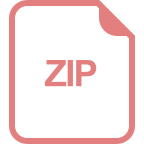
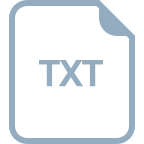



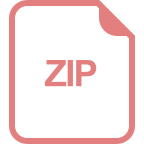