AttributeError: 'DataFrame' object has no attribute 'append'. Did you mean: '_append'?如何解决
时间: 2023-11-13 08:04:34 浏览: 145
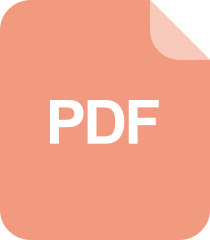
mdk_armcc_user_guide.pdf
AttributeError: 'DataFrame' object has no attribute 'append'错误通常是因为pandas版本更新导致的,新版本中已经将append方法替换为了concat方法。因此,如果你想要在DataFrame中添加新的行或列,可以使用concat方法。具体操作如下:
1.创建一个新的DataFrame,将要添加的行或列作为一个新的DataFrame。
2.使用concat方法将新的DataFrame与原始DataFrame合并。
下面是一个示例代码:
```
import pandas as pd
# 创建一个新的DataFrame
new_row = pd.DataFrame({'col1': [1, 2], 'col2': [3, 4]})
# 使用concat方法将新的DataFrame与原始DataFrame合并
df = pd.concat([df, new_row], axis=0)
```
在这个示例中,我们首先创建了一个新的DataFrame,然后使用concat方法将新的DataFrame与原始DataFrame合并。注意,我们使用了axis参数来指定我们要添加的是行还是列。如果要添加列,可以将axis参数设置为1。
阅读全文
相关推荐
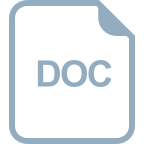
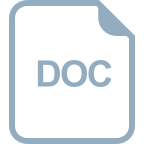










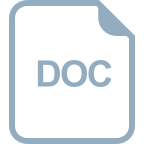
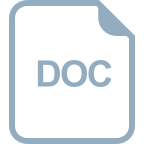
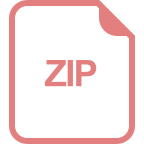
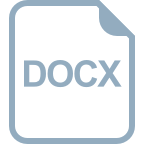
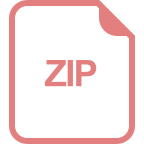