C(或C++)实现循环队列,主函数测试队空到队满,队满到队空的过程,要输出“入队”、“出队”、“队空”和“队满”的提示文字
时间: 2024-11-22 20:39:46 浏览: 3
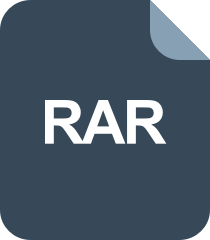
xunhuanduilie.rar_visual c_中断与duilie
在C语言中,我们可以创建一个循环队列的实现,它通过两个数组来模拟队列的行为,当一个队列满了,下一个数组开始作为队列的一部分。以下是循环队列的基本结构和操作:
```c
#include <stdio.h>
#include <stdlib.h>
#define QUEUE_SIZE 5
#define FRONT 0
#define REAR (FRONT + QUEUE_SIZE - 1)
typedef struct {
int* queue;
int rear;
int front;
} Queue;
// 初始化循环队列
Queue* createQueue() {
Queue* q = (Queue*)malloc(sizeof(Queue));
q->queue = (int*)malloc(QUEUE_SIZE * sizeof(int));
q->front = q->rear = -1;
return q;
}
// 检查队列是否为空
int isEmpty(Queue* q) {
return q->front == -1;
}
// 检查队列是否已满
int isFull(Queue* q) {
return (q->rear + 1) % QUEUE_SIZE == q->front;
}
// 入队操作
void enqueue(Queue* q, int value) {
if (isFull(q)) {
printf("Queue is full.\n");
} else {
q->rear = (q->rear + 1) % QUEUE_SIZE;
q->queue[q->rear] = value;
printf("Enqueue: %d\n", value);
}
}
// 出队操作
int dequeue(Queue* q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
} else {
int value = q->queue[q->front];
q->front = (q->front + 1) % QUEUE_SIZE;
printf("Dequeue: %d\n", value);
return value;
}
return -1; // 如果队列空,返回值表示无法出队
}
// 测试队空到队满,再到队空的过程
void testQueue(Queue* q) {
for (int i = 1; i <= QUEUE_SIZE * 2; ++i) {
if (i % QUEUE_SIZE == 0) { // 队满时出队
if (dequeue(q) != -1) {
printf("Dequeue after full: ");
}
} else { // 入队
enqueue(q, i);
}
printf("\nQueue status: ");
if (isEmpty(q)) {
printf("Empty.\n");
} else if (isFull(q)) {
printf("Full.\n");
} else {
printf("Not full or not empty.\n");
}
}
}
int main() {
Queue* queue = createQueue();
testQueue(queue);
free(queue->queue);
free(queue);
return 0;
}
```
这段代码首先定义了一个循环队列的数据结构,包括队列、队首和队尾指针。接着实现了一系列基本的操作,如检查队列状态、入队和出队。`testQueue`函数模拟了队空到队满的过程,并打印相应的提示信息。
阅读全文
相关推荐
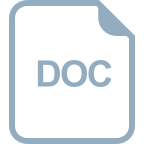
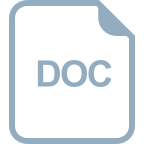









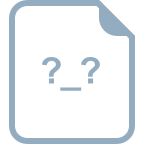





