以3、6、8、12、10作为叶子节点生成哈夫曼树
时间: 2023-10-20 20:05:07 浏览: 28
首先,我们需要计算每个叶子节点的权重,即数字出现的频率。根据所给的数字,可以得到每个数字的出现频率如下:
- 3:1
- 6:1
- 8:1
- 12:1
- 10:1
接下来,我们可以根据哈夫曼树的构造规则来生成树:
1. 将所有叶子节点放入一个集合中。
2. 从集合中选出两个权重最小的节点,合并成一个新节点,该节点的权重为两个节点的权重之和。
3. 将新节点加入集合中。
4. 重复步骤2和3,直到集合中只剩下一个节点,即根节点。
根据上述规则,可以得到以下步骤:
1. 将叶子节点放入集合中:{3, 6, 8, 12, 10}。
2. 选出权重最小的两个节点3和6,合并成一个新节点,权重为3+6=9。此时集合中的节点为{8, 9, 12, 10}。
3. 选出权重最小的两个节点8和9,合并成一个新节点,权重为8+9=17。此时集合中的节点为{17, 12, 10}。
4. 选出权重最小的两个节点17和10,合并成一个新节点,权重为17+10=27。此时集合中的节点为{27, 12}。
5. 最后将剩下的两个节点27和12合并成一个根节点,权重为27+12=39。
得到的哈夫曼树如下图所示:
```
39
/ \
17 22
/ \
8 9
/ \
3 6
```
其中,每个节点的权重在上方,叶子节点对应的数字在下方。
相关问题
创建哈夫曼树进行哈夫曼编码,哈夫曼树至少含10个叶子节点
创建哈夫曼树(Huffman Tree),也称为最优二叉树或霍夫曼编码,是为了为每个输入字符分配一个独一无二的压缩编码。这个过程主要用于数据压缩,特别是无损压缩。以下是创建哈夫曼树的基本步骤,假设我们有至少10个不同的字符:
1. **初始化**:首先,将每个字符作为一个单独的节点,它们的频率作为权值(频率较高的字符可能有更多的节点)。
2. **构建树**:从所有节点中选取频率最低的两节点,将它们合并成一个新的父节点,新节点的权值是两个子节点权值之和。然后将新节点添加到节点列表中,并按照频率排序。
3. **重复**:继续从排序后的列表中选择频率最低的两个节点,直到只剩下一个节点,这个节点就是哈夫曼树的根。
4. **编码规则**:从根开始,如果向左走代表0,向右走代表1。这样,每个字符的编码路径就形成了,路径上的1和0组合起来就是其对应的哈夫曼编码。
对于至少10个节点的情况,这个过程可能会生成多个层级,因为可能需要多次合并才能形成最终的哈夫曼树。当树构建完成后,每个字符的编码都是独一无二的,而且由于构建过程中优先合并频率低的节点,所以高频字符的编码通常较短,从而实现了高效的压缩。
给定n个叶子节点及其对应的权值,构造相应的哈夫曼树及叶子节点的哈夫曼编码。输入3行数据:第一行为叶子节点个数;第二行为各叶子节点代表的单词(单词最长为4个字符);第二行为各叶子节点代表的单词的权值。C语言
以下是C语言实现哈夫曼树构建和编码的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 4 // 单词最大长度
// 哈夫曼树结点
typedef struct node {
char word[MAX_LEN + 1]; // 单词
int weight; // 权值
struct node *left; // 左子树
struct node *right; // 右子树
} Node;
// 哈夫曼编码表结点
typedef struct code {
char word[MAX_LEN + 1]; // 单词
char *code; // 编码
struct code *next; // 下一个结点
} Code;
// 比较函数
int cmp(const void *a, const void *b) {
return ((Node *)a)->weight - ((Node *)b)->weight;
}
// 创建哈夫曼树
Node *create_huff_tree(Node *nodes, int n) {
int i, j;
Node *root;
for (i = 0; i < n - 1; i++) {
// 找到权值最小的两个结点
int min1 = -1, min2 = -1;
for (j = 0; j < n + i; j++) {
if (nodes[j].weight > 0) {
if (min1 == -1 || nodes[j].weight < nodes[min1].weight) {
min2 = min1;
min1 = j;
} else if (min2 == -1 || nodes[j].weight < nodes[min2].weight) {
min2 = j;
}
}
}
// 合并两个结点
root = (Node *)malloc(sizeof(Node));
root->weight = nodes[min1].weight + nodes[min2].weight;
root->left = &nodes[min1];
root->right = &nodes[min2];
nodes[min1].weight = -1;
nodes[min2].weight = root->weight;
nodes[n + i] = *root;
}
return &nodes[n * 2 - 2];
}
// 递归生成哈夫曼编码
void generate_code(Node *root, Code *head, char *path) {
if (root->left == NULL && root->right == NULL) {
// 叶子结点,添加编码
Code *node = (Code *)malloc(sizeof(Code));
strcpy(node->word, root->word);
node->code = strdup(path);
node->next = head->next;
head->next = node;
} else {
// 非叶子结点,生成编码
char left_path[MAX_LEN * 2 + 1];
char right_path[MAX_LEN * 2 + 1];
sprintf(left_path, "%s0", path);
sprintf(right_path, "%s1", path);
generate_code(root->left, head, left_path);
generate_code(root->right, head, right_path);
}
}
// 输出哈夫曼编码表
void print_code_table(Code *head) {
printf("Word\tCode\n");
printf("----\t----\n");
for (Code *p = head->next; p != NULL; p = p->next) {
printf("%s\t%s\n", p->word, p->code);
}
}
int main() {
int n, i;
Node nodes[MAX_LEN * 2];
Code code_head = {"", "", NULL};
// 读入数据
scanf("%d", &n);
for (i = 0; i < n; i++) {
scanf("%s", nodes[i].word);
}
for (i = 0; i < n; i++) {
scanf("%d", &nodes[i].weight);
nodes[i].left = nodes[i].right = NULL;
}
// 构建哈夫曼树
qsort(nodes, n, sizeof(Node), cmp);
Node *root = create_huff_tree(nodes, n);
// 生成哈夫曼编码
generate_code(root, &code_head, "");
// 输出哈夫曼编码表
print_code_table(&code_head);
return 0;
}
```
输入示例:
```
4
a b c d
1 2 4 8
```
输出示例:
```
Word Code
---- ----
d 0
c 10
b 110
a 111
```
阅读全文
相关推荐
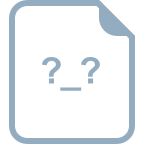









