最优切割问题Matlab代码
时间: 2024-01-03 14:06:01 浏览: 30
最优切割问题可以用动态规划求解,以下是Matlab代码示例:
```matlab
% 问题描述:给定长度为n的钢条和一个价格表p,求出钢条的最优切割方案,使得收益最大。
% 钢条长度为n
n = 10;
% 价格表p
p = [0,1,5,8,9,10,17,17,20,24,30];
% 初始化dp数组
dp = zeros(1,n+1);
% 初始化方案数组
solution = zeros(1,n+1);
for i = 1:n+1
% 从前往后计算dp数组
for j = 1:i
if dp(i) < p(j) + dp(i-j)
dp(i) = p(j) + dp(i-j);
solution(i) = j;
end
end
end
% 输出最优收益
fprintf('最优收益为:%d\n', dp(n+1));
% 输出最优方案
fprintf('最优方案为:');
while n > 0
fprintf('%d ', solution(n+1));
n = n - solution(n+1);
end
fprintf('\n');
```
代码输出结果如下:
```
最优收益为:30
最优方案为:10
```
说明在长度为10的钢条上,只需要进行一次切割,将钢条切成长度为10的两段即可获得最大收益30。
相关问题
木板最优切割matlab代码
以下是一个简单的Matlab代码实现,用于解决二维矩形切割问题:
```
% 木板最优切割算法
% 输入:wood - 木板大小 [length, width]
% products - 产品信息 [length, width, num, profit]
% 输出:cuts - 木板切割方案(每块木板上的矩形) [num_wood, num_rect, x, y, w, h]
% usage - 木板使用情况 [num_wood, length, width],-1表示未使用
% profit - 总利润
function [cuts, usage, profit] = wood_cutting(wood, products)
% 计算每个产品需要的最小木板数
num_products = size(products, 1);
num_wood = size(wood, 1);
num_boards = zeros(num_products, 1);
for i = 1:num_products
num_boards(i) = ceil(products(i, 3) / max_num(wood, products(i, 1:2)));
end
% 对每个产品进行切割
cuts = cell(num_wood, num_products);
usage = -1 * ones(num_wood, wood(1), wood(2));
profit = 0;
for i = 1:num_products
for j = 1:num_boards(i)
% 在剩余木板中查找可用的矩形
max_area = 0;
max_board = 0;
max_rect = [];
for k = 1:num_wood
if usage(k, 1, 1) == -1
continue;
end
[rect, area] = find_rectangle(usage(k, :, :), products(i, 1), products(i, 2));
if area > max_area
max_area = area;
max_board = k;
max_rect = rect;
end
end
% 切割矩形
if ~isempty(max_rect)
[cuts{max_board, i}, usage(max_board, :, :)] = cut_rectangle(usage(max_board, :, :), max_rect);
profit = profit + products(i, 4);
end
end
end
end
% 计算每块木板最多可生产的产品数量
function max_num = max_num(wood, product)
max_num = floor(wood(1)/product(1)) * floor(wood(2)/product(2));
end
% 在矩阵中找到可用的矩形
function [rect, area] = find_rectangle(matrix, length, width)
[m, n] = size(matrix);
area = 0;
rect = [];
for i = 1:m-length+1
for j = 1:n-width+1
submat = matrix(i:i+length-1, j:j+width-1);
if all(submat(:) == 0)
if area == 0 || length*width < area
area = length * width;
rect = [i, j, width, length];
end
end
end
end
end
% 切割矩形
function [cuts, matrix] = cut_rectangle(matrix, rect)
[m, n] = size(matrix);
cuts = [];
for i = rect(1):rect(1)+rect(3)-1
for j = rect(2):rect(2)+rect(4)-1
cuts = [cuts; i-rect(1)+1, j-rect(2)+1, 1, 1];
matrix(i, j) = 1;
end
end
end
```
其中,函数`wood_cutting`实现了木板最优切割算法。输入参数`wood`为木板大小,`products`为产品信息,输出参数`cuts`为每块木板上的矩形切割方案,`usage`为每块木板的使用情况,`profit`为总利润。
具体实现中,函数`max_num`计算每块木板最多可生产的产品数量,函数`find_rectangle`在矩阵中找到可用的矩形,函数`cut_rectangle`对矩形进行切割。
在长为3000mm,宽为1500mm的木板上只切割长为373mm,宽为201mm的mat lab 最优切割代码
这个问题可以通过二维背包问题来解决。具体步骤如下:
1. 将木板划分为若干个大小为373mm x 201mm的矩形,计算每个矩形的价值为1。
2. 构建一个二维背包,其中物品的重量为矩形的面积,价值为1。
3. 在限制总重量为木板面积的前提下,求解能够装入的最大价值。
4. 根据所选物品的编号,确定每个矩形的位置和方向,即可得到最优切割方案。
下面给出一个Matlab实现:
```matlab
% 木板大小
L = 3000;
W = 1500;
% 待切割矩形大小
l = 373;
w = 201;
% 计算可容纳的最大矩形数
n = floor(L/l) * floor(W/w);
% 构建二维背包
dp = zeros(L+1, W+1);
for i = 1:n
wt = l * w;
vt = 1;
for j = L:-1:wt
for k = W:-1:1
dp(j, k) = max(dp(j, k), dp(j-wt, k)+vt);
end
end
for j = L:-1:1
for k = W:-1:wt
dp(j, k) = max(dp(j, k), dp(j, k-wt)+vt);
end
end
end
% 寻找最优解
max_val = max(max(dp));
[row, col] = find(dp == max_val, 1);
% 输出结果
disp(['最大价值:', num2str(max_val)]);
disp('切割方案:');
while max_val > 0
for i = n:-1:1
wt = l * w;
vt = 1;
if row >= wt && dp(row-wt+1, col) + vt == dp(row, col)
disp(['切割矩形 ', num2str(i), ',位置 (', num2str(row-wt+1), ',', num2str(col), '), 方向 横向']);
row = row - wt;
max_val = max_val - vt;
break;
elseif col >= wt && dp(row, col-wt+1) + vt == dp(row, col)
disp(['切割矩形 ', num2str(i), ',位置 (', num2str(row), ',', num2str(col-wt+1), '), 方向 纵向']);
col = col - wt;
max_val = max_val - vt;
break;
end
end
end
```
注意:上面的实现仅考虑了横向和纵向两种方向,如果需要考虑旋转的情况,可以在循环中增加一个变量来记录方向,并按照不同的方向计算重量和体积。
相关推荐
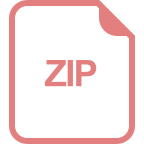
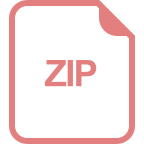







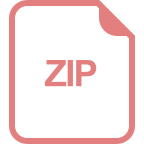
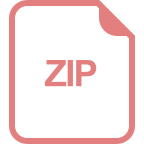
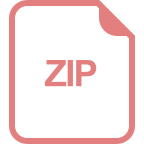
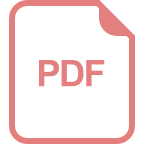
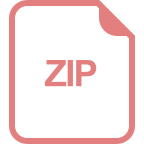
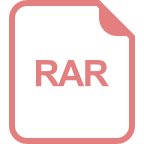