帮我写一个C语言代码用堆栈实现加减乘除求值
时间: 2024-05-19 16:15:34 浏览: 62
好的,以下是使用堆栈实现加减乘除求值的C语言代码:
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int top;
int data[MAX_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
void push(Stack *s, int x) {
if (s->top >= MAX_SIZE - 1) {
printf("Stack overflow!\n");
exit(-1);
}
s->data[++(s->top)] = x;
}
int pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(-1);
}
return s->data[(s->top)--];
}
int main() {
Stack s;
init(&s);
char ch;
int num1, num2, result;
while ((ch = getchar()) != '\n') {
if (ch >= '0' && ch <= '9') {
// 数字入栈
num1 = ch - '0';
push(&s, num1);
} else {
// 进行运算
num2 = pop(&s);
num1 = pop(&s);
switch (ch) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
printf("Invalid operator\n");
exit(-1);
}
// 运算结果入栈
push(&s, result);
}
}
printf("Result: %d\n", pop(&s));
return 0;
}
希望能对您有所帮助!
阅读全文
相关推荐
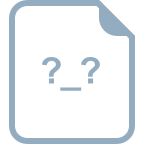
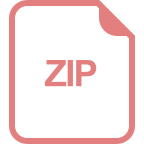
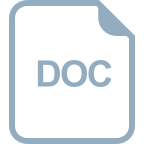
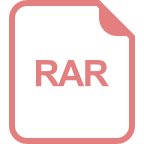



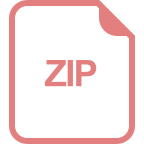
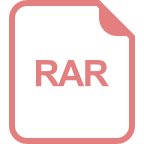
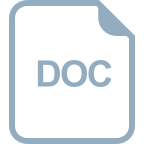
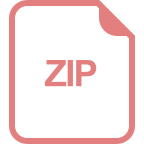
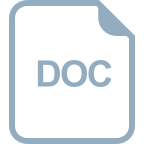
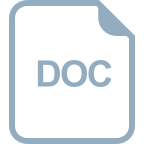


