inputs = tf.keras.Input((height, width, 1)) x = inputs # Multi-Wiener deconvol utions x = WienerDeconvolution(initial_psf, initial_K)(x) skips = [] # Contracting path for c in encoding_cs: x, x_skip = encoder_block(x, c, kernel_size=3, padding='same', dilation_rate=1, pooling='average') skips.append(x_skip) skips = list(reversed(skips)) # Center x = conv2d_block(x, center_cs, kernel_size=3, padding='same') # Expansive path for i, c in enumerate(decoding_cs): if skip_connections[i]: x = decoder_block_resize(x, skips[i], c, kernel_size=3, padding='same', dilation_rate=1) else: x = decoder_block(x, None, c, kernel_size=3, padding='same', dilation_rate=1) # Classify x = layers.Conv2D(filters=1, kernel_size=1, use_bias=True, activation='relu')(x) outputs = tf.squeeze(x, axis=3) model = tf.keras.Model(inputs=[inputs], outputs=[outputs])
时间: 2023-12-08 13:04:23 浏览: 40
这段代码是一个基于深度学习的图像去模糊模型,使用了U-Net的结构。代码中包含了两个子模块:编码器和解码器。编码器通过多个卷积层将输入图像压缩为特征图,其中的池化操作可以缩小特征图的尺寸。在编码器的每一层中,都会保存一个跳跃连接,将该层的特征图保存下来,以便在解码器中恢复图像细节时使用。解码器则将特征图进行上采样,逐渐恢复图像细节,每一层都会将对应编码器层的跳跃连接特征图进行拼接,以恢复更多的细节。最后,使用一个卷积层将输出压缩为一维,得到最终的去模糊结果。
相关问题
input_layer = tf.keras.layers.Input(shape=inputs.shape[1:])是什么意思
`tf.keras.layers.Input`是一个Keras层,用于接收模型的输入数据。这里,我们使用`tf.keras.layers.Input`来构建输入层,其中`shape`参数表示输入数据的形状。
`inputs.shape[1:]`表示输入数据的形状,其中`inputs`是输入数据的张量。`inputs.shape`是一个元组,包含了输入数据的形状信息,如`(batch_size, input_dim)`。`inputs.shape[1:]`则表示除了`batch_size`之外的其它维度,也就是`input_dim`。
因此,`tf.keras.layers.Input(shape=inputs.shape[1:])`的意思是:创建一个输入层,其输入数据的形状与`inputs`张量的形状相同,但去掉了`batch_size`这一维。这样做的好处是,可以在训练时灵活地调整`batch_size`的大小,而不必每次都修改模型的输入层。
修改下面代码使其具体生成器的卷积lstm更换为卷积RNN def build_generator(self): def res_net(inputs, filters): x = inputs net = conv2d(x, filters // 2, (1, 1), 1) net = conv2d(net, filters, (3, 3), 1) net = net + x # net=tf.keras.layers.LeakyReLU(0.2)(net) return net def conv2d(inputs, filters, kernel_size, strides): x = tf.keras.layers.Conv2D(filters, kernel_size, strides, 'same')(inputs) x = tf.keras.layers.BatchNormalization()(x) x = tf.keras.layers.LeakyReLU(alpha=0.2)(x) return x d0 = tf.keras.layers.Input(shape=(10, 128, 128, 3)) out= tf.keras.layers.ConvLSTM2D(filters=32, kernel_size=3,padding='same')(d0) out=tf.keras.layers.Conv2D(3,1,1,'same')(out) return keras.Model(inputs=d0, outputs=out)
修改如下:
```python
def build_generator(self):
def res_net(inputs, filters):
x = inputs
net = conv2d(x, filters // 2, (1, 1), 1)
net = conv2d(net, filters, (3, 3), 1)
net = net + x
return net
def conv2d(inputs, filters, kernel_size, strides):
x = tf.keras.layers.Conv2D(filters, kernel_size, strides, 'same')(inputs)
x = tf.keras.layers.BatchNormalization()(x)
x = tf.keras.layers.LeakyReLU(alpha=0.2)(x)
return x
d0 = tf.keras.layers.Input(shape=(10, 128, 128, 3))
out = tf.keras.layers.ConvRNN2D(filters=32, kernel_size=3, padding='same')(d0)
out = tf.keras.layers.Conv2D(3, 1, 1, 'same')(out)
return keras.Model(inputs=d0, outputs=out)
```
将 `ConvLSTM2D` 替换为 `ConvRNN2D` 即可,它们的参数意义和使用方法都是一样的。
相关推荐
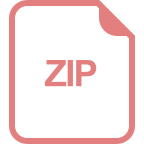
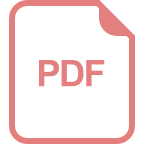












