x = tf.keras. Input( shape=(n_inputs, ) ,dtype=tf.float32)
时间: 2024-05-26 16:18:37 浏览: 19
这段代码是在 TensorFlow 中创建一个输入层,其中:
- `tf.keras` 是 TensorFlow 中的高级 API,提供了方便的模型构建和训练功能。
- `Input` 函数用于创建一个输入层,其参数包括输入数据的形状 (`shape`) 和数据类型 (`dtype`)。
- `shape=(n_inputs,)` 表示输入数据的形状是一个长度为 `n_inputs` 的一维数组。
- `dtype=tf.float32` 表示输入数据的数据类型是 `float32`。
相关问题
tf.keras.Input
### 回答1:
`tf.keras.Input`是一个用于定义模型输入的函数,它可以用于构建基于Keras的深度学习模型。它的作用是将输入数据的形状(shape)传递给模型,并给这个输入数据一个可读性强的名称。在使用`tf.keras.Input`函数时,需要指定输入数据的形状、数据类型和名称等参数。例如,下面的代码定义了一个输入形状为(32, 32, 3)的float32类型的张量,并命名为`input_tensor`:
```python
import tensorflow as tf
input_tensor = tf.keras.Input(shape=(32, 32, 3), dtype=tf.float32, name='input_tensor')
```
在模型构建时,可以使用这个`input_tensor`作为模型的输入。例如,下面的代码构建了一个简单的全连接神经网络模型:
```python
x = tf.keras.layers.Flatten()(input_tensor)
x = tf.keras.layers.Dense(128, activation='relu')(x)
output_tensor = tf.keras.layers.Dense(10, activation='softmax')(x)
model = tf.keras.Model(inputs=input_tensor, outputs=output_tensor)
```
这个模型的输入是`input_tensor`,输出是`output_tensor`。
### 回答2:
tf.keras.Input是TensorFlow中的一个函数,用于定义模型的输入层。
在使用tf.keras建立神经网络模型时,首先我们需要定义模型的输入。tf.keras.Input函数的作用就是为我们提供了一个方便的方法来定义模型的输入层。
它的用法如下:
input = tf.keras.Input(shape=(input_shape,))
其中,shape参数指定了输入层的形状。
具体来说,shape参数是一个元组,描述了输入张量的维度。例如,shape=(32, 32, 3)表示输入张量是一个三维张量,其中宽度为32,高度为32,通道数为3。
通过tf.keras.Input函数定义的输入层本质上是一个占位符,它没有实际的数值,只是用来占位。在模型训练时,我们会利用训练数据填充这个输入层。
在定义完输入层后,我们可以将输入层与其他层连接,构建一个完整的神经网络模型。例如:
x = tf.keras.Conv2D(32, (3,3))(input)
这行代码表示将输入层与一个卷积层连接起来,其中卷积层的输出是一个32通道的特征图。
通过tf.keras.Input函数定义的输入层是通过函数式API的方式定义模型的输入。相比于Sequential模型,函数式API更加灵活,可以处理更加复杂的模型结构。
总之,tf.keras.Input函数是TensorFlow中用来定义模型输入层的一个函数,它通过shape参数来指定输入张量的形状,然后与其他层连接构建一个完整的神经网络模型。
### 回答3:
tf.keras.Input是TensorFlow中的一个函数,用于定义Keras模型的输入层。
在Keras中,每个模型都必须有一个输入层。输入层是模型的起点,它接收输入数据,并根据指定的形状将数据转换为张量。tf.keras.Input函数就是用来定义这个输入层的。
tf.keras.Input函数有以下参数:
- shape:指定输入数据的形状。它是一个元组或列表,表示输入数据的每个维度的大小。例如,shape=(32,32,3)表示输入数据是一个32x32像素的RGB图像。
- dtype:指定输入数据的数据类型。默认为float32。
- sparse:指定输入是否稀疏。当输入数据是一个稀疏矩阵时,将sparse参数设置为True。默认为False。
- name:给输入层命名的字符串。
使用tf.keras.Input函数定义输入层后,我们可以将其作为Keras模型的第一层。例如:
```
input_layer = tf.keras.Input(shape=(32,32,3))
```
定义完输入层后,我们可以使用它作为其他层的输入,通过层与层之间的连接来构建模型。
通过tf.keras.Input函数定义输入层的好处是,我们可以更方便地将输入与其他层连接起来,而不需要明确地指定输入的形状和数据类型。Keras会根据输入层的定义自动识别并应用正确的转换。
总之,tf.keras.Input是TensorFlow中用于定义Keras模型的输入层的函数,它可以方便地定义输入的形状和数据类型,并作为模型的起点,连接其他的层构建整个模型。
input_image = tf.placeholder("float", [None, 80, 100, 4]) # 游戏像素 action = tf.placeholder("float", [None, output]) # 操作用TensorFlow2代码改写
在TensorFlow 2中,可以使用`tf.keras.Input`创建输入占位符,例如:
```
import tensorflow as tf
# 创建输入占位符
input_image = tf.keras.Input(shape=(80, 100, 4), dtype=tf.float32)
action = tf.keras.Input(shape=(output,), dtype=tf.float32)
```
这里使用`tf.keras.Input`函数来创建输入占位符,其中`shape`参数指定输入的形状,`dtype`参数指定输入的数据类型。注意,这里的输入占位符不再是`tf.placeholder`类型,而是`tf.Tensor`类型。
在使用这些占位符进行计算时,可以直接将它们传递给`tf.keras.Model`的`__call__`方法,例如:
```
import tensorflow as tf
# 创建输入占位符
input_image = tf.keras.Input(shape=(80, 100, 4), dtype=tf.float32)
action = tf.keras.Input(shape=(output,), dtype=tf.float32)
# 构建模型
x = tf.keras.layers.Conv2D(filters=32, kernel_size=3)(input_image)
x = tf.keras.layers.MaxPooling2D()(x)
x = tf.keras.layers.Flatten()(x)
x = tf.keras.layers.Dense(units=64, activation='relu')(x)
output = tf.keras.layers.Dense(units=output, activation='softmax')(x)
model = tf.keras.Model(inputs=[input_image, action], outputs=output)
# 使用输入占位符进行计算
input_image_data = tf.ones((32, 80, 100, 4))
action_data = tf.ones((32, output))
output_data = model([input_image_data, action_data])
```
这里使用`Model`类构建一个简单的神经网络模型,并将输入和输出占位符作为构造函数的参数传递给模型。然后,可以使用`model`对象调用模型进行计算,传递输入数据即可。
相关推荐
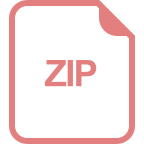
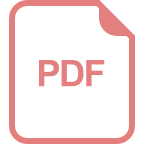
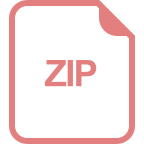












