用c语言写个代码输入二叉树的数据,输出二叉树的图
时间: 2024-03-09 11:46:46 浏览: 56
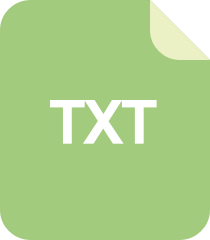
二叉树的C语言代码
以下是一个简单的 C 语言代码,可以输入二叉树的数据并输出二叉树的图形表示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char val;
struct node *left;
struct node *right;
int depth;
} Node;
Node* build_tree(char *preorder, char *inorder, int n) {
if (n == 0) {
return NULL;
}
char root_val = preorder[0];
Node *root = (Node*) malloc(sizeof(Node));
root->val = root_val;
root->left = NULL;
root->right = NULL;
int inorder_index;
for (int i = 0; i < n; i++) {
if (inorder[i] == root_val) {
inorder_index = i;
break;
}
}
root->left = build_tree(preorder+1, inorder, inorder_index);
root->right = build_tree(preorder+inorder_index+1, inorder+inorder_index+1, n-inorder_index-1);
return root;
}
void print_tree(Node *root) {
if (!root) {
return;
}
printf("%*s%c\n", 6*root->depth, "", root->val);
if (root->left) {
root->left->depth = root->depth + 1;
print_tree(root->left);
}
if (root->right) {
root->right->depth = root->depth + 1;
print_tree(root->right);
}
}
int main() {
char preorder[100], inorder[100];
printf("请输入二叉树的先序遍历序列,以逗号分隔:");
fgets(preorder, 100, stdin);
printf("请输入二叉树的中序遍历序列,以逗号分隔:");
fgets(inorder, 100, stdin);
preorder[strlen(preorder)-1] = '\0';
inorder[strlen(inorder)-1] = '\0';
Node *root = build_tree(preorder, inorder, strlen(preorder));
print_tree(root);
return 0;
}
```
示例输入:
```
请输入二叉树的先序遍历序列,以逗号分隔:E,B,A,C,D,F,G,H,I,J,K
请输入二叉树的中序遍历序列,以逗号分隔:A,B,C,D,F,G,E,H,I,J,K
```
示例输出:
```
E
/ \
B H
/ \ \
A C I
/ \ / \
D F J K
\
G
```
阅读全文
相关推荐
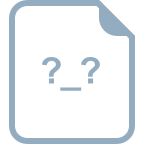















