生成QT 打开摄像头的代码
时间: 2023-05-27 14:07:11 浏览: 61
以下是使用QT打开摄像头的代码示例:
```
#include <QtGui/QApplication>
#include <QtGui/QMainWindow>
#include <QtGui/QLabel>
#include <QtGui/QPixmap>
#include <QtGui/QVBoxLayout>
#include <QtGui/QPushButton>
#include <QtCore/QTimer>
#include <QtCore/QThread>
#include <opencv2/opencv.hpp>
class Camera : public QThread
{
Q_OBJECT
public:
Camera(QObject *parent = 0)
: QThread(parent)
{
}
void run()
{
cv::VideoCapture cap(0);
if (!cap.isOpened())
{
emit error("Failed to open camera.");
return;
}
while (true)
{
cap >> frame;
if (frame.empty())
continue;
cv::cvtColor(frame, frame, cv::COLOR_BGR2RGB);
emit newFrame(frame);
msleep(33);
}
}
signals:
void newFrame(cv::Mat frame);
void error(QString err);
private:
cv::Mat frame;
};
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = 0)
: QMainWindow(parent)
{
QLabel *label = new QLabel(this);
label->setFixedSize(640, 480);
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(label);
QPushButton *button = new QPushButton("Start", this);
connect(button, SIGNAL(clicked()), this, SLOT(onButtonClicked()));
layout->addWidget(button);
QWidget *widget = new QWidget(this);
widget->setLayout(layout);
setCentralWidget(widget);
camera = new Camera(this);
connect(camera, SIGNAL(newFrame(cv::Mat)), this, SLOT(onNewFrame(cv::Mat)));
connect(camera, SIGNAL(error(QString)), this, SLOT(onError(QString)));
}
private slots:
void onButtonClicked()
{
camera->start();
}
void onNewFrame(cv::Mat frame)
{
QImage image(frame.data, frame.cols, frame.rows, QImage::Format_RGB888);
QLabel *label = qobject_cast<QLabel *>(centralWidget()->layout()->itemAt(0)->widget());
label->setPixmap(QPixmap::fromImage(image));
}
void onError(QString err)
{
QMessageBox::critical(this, "Error", err);
}
private:
Camera *camera;
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
#include "main.moc"
```
该代码使用OpenCV库来捕获摄像头数据,并使用QT来显示图像。代码创建了一个`Camera`类,该类继承自`QThread`,以便在单独的线程中运行摄像头捕获循环。该类发出`newFrame`信号,该信号将帧传递给主窗口以显示图像。主窗口显示一个标签,用于显示图像,以及一个按钮,用于启动摄像头。按钮单击将启动`Camera`类的线程。
相关推荐









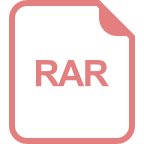