编程实现图像变暗,图像变亮,降低对比度,gamma变换代码
时间: 2024-09-19 09:02:35 浏览: 42
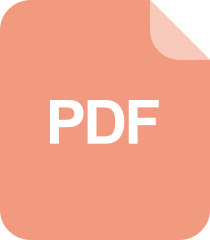
浅谈Python Opencv中gamma变换的使用详解
在编程中,特别是Python中,可以使用PIL(Pillow)库来进行图像处理。以下是一些基本操作的代码示例:
1. 图像变暗 (Brightness Decrease):
```python
from PIL import Image, ImageEnhance
def darken_image(image_path, factor=0.5):
img = Image.open(image_path)
enhancer = ImageEnhance.Brightness(img)
dark_img = enhancer.enhance(factor)
return dark_img.save('darkened_' + image_path)
# 使用
darken_image('original.jpg')
```
2. 图像变亮 (Brightness Increase):
```python
def brighten_image(image_path, factor=1.5):
# ... 相同的过程,只是enhance函数传入的是factor大于1的值
enhancer = ImageEnhance.Brightness(img)
bright_img = enhancer.enhance(factor)
return bright_img.save('brightened_' + image_path)
```
3. 降低对比度 (Reduce Contrast):
```python
def reduce_contrast(image_path, factor=0.5):
img = Image.open(image_path)
enhancer = ImageEnhance.Contrast(img)
low_contrast_img = enhancer.enhance(factor)
return low_contrast_img.save('low_contrast_' + image_path)
```
4. Gamma变换 (Non-linear contrast adjustment):
```python
import cv2
import numpy as np
def gamma_correction(image_path, gamma=0.5):
img = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
inv_gamma = 1.0 / gamma
table = np.array([((i / 255.0) ** inv_gamma) * 255 for i in np.arange(0, 256)]).astype("uint8")
corrected_img = cv2.LUT(img, table)
return cv2.imwrite('corrected_' + image_path, corrected_img)
```
每个函数都需要对应的图片文件路径,并可以根据需要调整因素值。
阅读全文
相关推荐
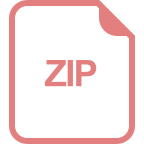
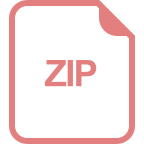





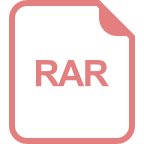
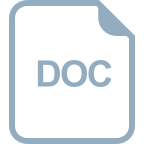
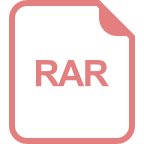
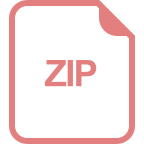
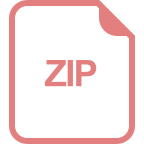
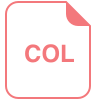
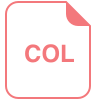



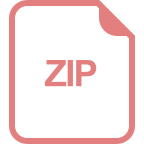