用python写图神经网络代码,要求输入和输出
时间: 2024-03-07 20:48:16 浏览: 20
好的,下面是一个简单的图神经网络代码示例,它使用 PyTorch 实现,输入为节点特征和邻接矩阵,输出为每个节点的类别预测结果。
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class GraphConvolution(nn.Module):
def __init__(self, in_features, out_features):
super(GraphConvolution, self).__init__()
self.weight = nn.Parameter(torch.FloatTensor(in_features, out_features))
self.bias = nn.Parameter(torch.FloatTensor(out_features))
def forward(self, x, adj):
support = torch.mm(x, self.weight)
output = torch.spmm(adj, support)
output = output + self.bias
return output
class GCN(nn.Module):
def __init__(self, nfeat, nhid, nclass):
super(GCN, self).__init__()
self.gc1 = GraphConvolution(nfeat, nhid)
self.gc2 = GraphConvolution(nhid, nclass)
def forward(self, x, adj):
x = F.relu(self.gc1(x, adj))
x = self.gc2(x, adj)
return F.log_softmax(x, dim=1)
# 输入参数
nfeat = 10 # 节点特征的维度
nhid = 16 # 隐层特征的维度
nclass = 2 # 输出的类别数目
adj = torch.FloatTensor([[0, 1, 1], [1, 0, 1], [1, 1, 0]]) # 邻接矩阵
x = torch.randn(3, nfeat) # 节点特征
# 创建模型
model = GCN(nfeat, nhid, nclass)
# 前向传播
output = model(x, adj)
print(output)
```
在上面的代码中,`GraphConvolution` 类实现了图卷积层,`GCN` 类实现了一个简单的图卷积网络。在输入参数中,`nfeat` 表示节点特征的维度,`nhid` 表示隐层特征的维度,`nclass` 表示输出的类别数目,`adj` 表示邻接矩阵,`x` 表示节点特征。在模型的前向传播过程中,先通过 `GraphConvolution` 类实现图卷积层的计算,然后使用 ReLU 激活函数进行非线性变换,最后通过 `GCN` 类实现输出层的计算,使用 log_softmax 函数进行输出结果的归一化。
相关推荐
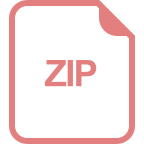
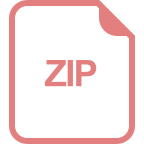
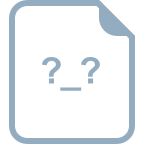














