serverSocketChannel.socket().bind(new InetSocketAddress(port));是什么意思
时间: 2024-05-19 09:15:15 浏览: 132
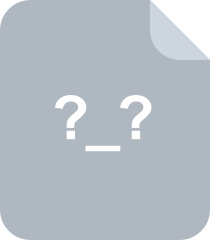
服务器socket连接
这个代码是在Java中建立一个服务器,指定服务器运行的端口号是port,并绑定到一个本地地址上。具体来说,它创建一个ServerSocketChannel实例,然后使用socket()方法获取一个ServerSocket对象,最后使用bind()方法将端口绑定到这个ServerSocket对象上。这样客户端就可以通过这个端口与服务器通信了。
阅读全文
相关推荐
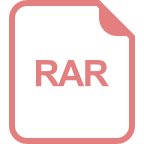
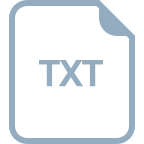
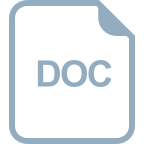
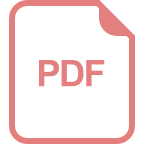
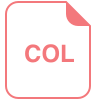
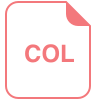
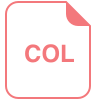
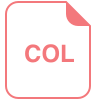
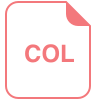
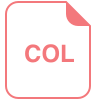
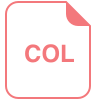
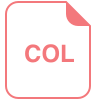
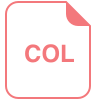
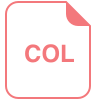
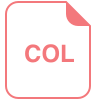


