Java网络编程实战:Socket、NIO、Netty,构建高效网络应用
发布时间: 2024-05-23 19:19:08 阅读量: 11 订阅数: 20 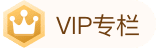
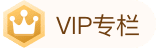
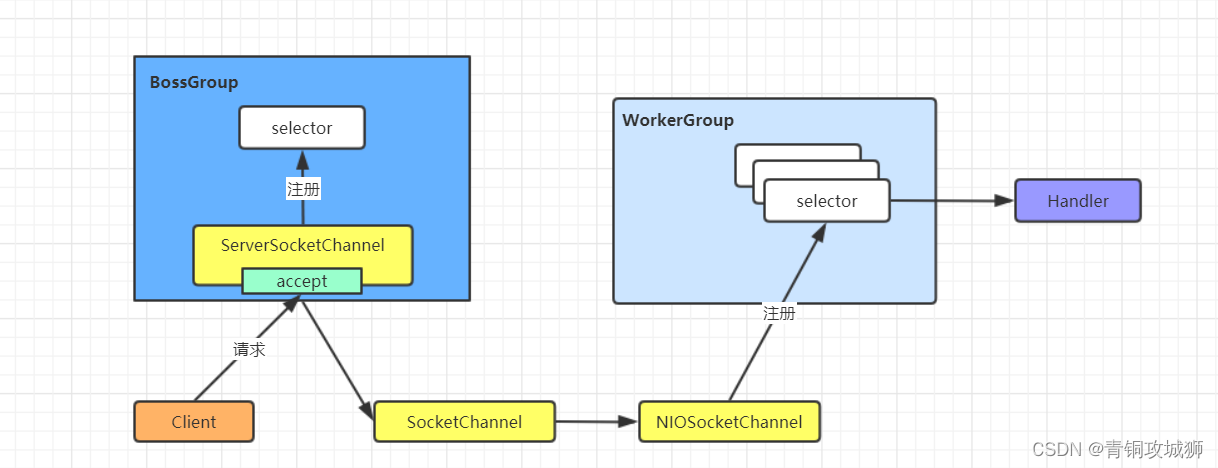
# 1. Java网络编程基础**
Java网络编程是利用Java语言开发网络应用程序的基础。本章将介绍Java网络编程的基础知识,包括:
- **网络编程的概念:**网络编程的定义、目的和应用场景。
- **网络模型:**介绍客户端-服务器模型、对等模型和分布式模型,并分析其优缺点。
- **网络协议:**介绍TCP/IP协议栈,重点讲解TCP和UDP协议的特性和区别。
# 2. Socket编程实战**
**2.1 Socket编程基础**
**2.1.1 Socket的概念和类型**
Socket是一种网络编程接口,它允许应用程序通过网络与其他计算机进行通信。Socket可以分为两类:
- **流式Socket:**用于传输连续的数据流,如文本、图像或视频。
- **数据报Socket:**用于传输离散的数据包,如网络数据包或电子邮件。
**2.1.2 Socket的创建和连接**
要创建Socket,需要使用`java.net.Socket`类。该类提供了一个构造函数,用于指定目标主机和端口号。
```java
Socket socket = new Socket("localhost", 8080);
```
创建Socket后,可以使用`connect()`方法将其连接到远程主机。
```java
socket.connect(new InetSocketAddress("localhost", 8080));
```
**2.2 Socket通信协议**
Socket编程使用两种主要的通信协议:
**2.2.1 TCP协议**
TCP(传输控制协议)是一种面向连接的协议,它提供可靠、有序的数据传输。TCP建立连接后,会维护一个状态机,以确保数据按顺序传输,并且不会丢失或损坏。
**2.2.2 UDP协议**
UDP(用户数据报协议)是一种无连接的协议,它提供不可靠、无序的数据传输。UDP不会建立连接,而是直接将数据包发送到目标主机。UDP适用于不需要可靠性或顺序传输的应用,如视频流或网络游戏。
**2.3 Socket编程实例**
**2.3.1 客户端-服务器通信**
以下是使用Socket进行客户端-服务器通信的示例:
**客户端代码:**
```java
Socket socket = new Socket("localhost", 8080);
OutputStreamWriter writer = new OutputStreamWriter(socket.getOutputStream());
writer.write("Hello from client!");
writer.flush();
```
**服务器代码:**
```java
ServerSocket serverSocket = new ServerSocket(8080);
Socket clientSocket = serverSocket.accept();
InputStreamReader reader = new InputStreamReader(clientSocket.getInputStream());
BufferedReader bufferedReader = new BufferedReader(reader);
String message = bufferedReader.readLine();
System.out.println("Received message from client: " + message);
```
**2.3.2 多线程并发编程**
为了处理多个客户端连接,可以使用多线程并发编程。以下示例使用线程池来处理客户端请求:
```java
ExecutorService executorService = Executors.newFixedThreadPool(10);
ServerSocket serverSocket = new ServerSocket(8080);
while (true) {
Socket clientSocket = serverSocket.accept();
executorService.submit(() -> {
// 处理客户端请求
});
}
```
# 3. NIO编程实战
### 3.1 NIO编程基础
#### 3.1.1 NIO的概念和优势
NIO(Non-Blocking IO)是一种非阻塞式的IO模型,它与传统的阻塞式IO模型相比,具有以下优势:
- **非阻塞:** NIO不会阻塞线程,即使在等待IO操作完成时也是如此。这使得NIO可以处理大量的并发连接,而不会导致线程饥饿。
- **高性能:** NIO使用缓冲区和选择器来高效地管理IO操作,从而提高了应用程序的性能。
- **可扩展性:** NIO可以轻松地扩展到处理大量的并发连接,因为它不会阻塞线程。
#### 3.1.2 NIO的缓冲区和选择器
NIO使用缓冲区和选择器来管理IO操作。缓冲区用于存储数据,而选择器用于监控多个IO通道的状态。
**缓冲区:** 缓冲区是用于存储数据的内存区域。NIO使用两种类型的缓冲区:
- **直接缓冲区:** 直接缓冲区直接映射到物理内存,从而可以更有效地访问数据。
- **非直接缓冲区:** 非直接缓冲区将数据复制到JVM堆中,然后再进行访问。
**选择器:** 选择器是一个Java类,它允许应用程序同时监控多个IO通道。选择器可以检测以下事件:
- **读事件:** 通道中有数据可读。
- **写事件:** 通道可以写入数据。
- **连接事件:** 通道已连接或断开连接。
### 3.2 NIO编程实例
#### 3.2.1 Echo服务器
以下是一个使用NIO实现的Echo服务器示例:
```java
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
import java.util.Set;
public class EchoServer {
private Selector selector;
private ServerSocketChannel serverSocketChannel;
public EchoServer(int port) throws IOException {
selector = Selector.open();
serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.bind(new InetSocketAddress(port));
serverSocketChannel.configureBlocking(false);
serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT);
}
public void start() throws IOException {
while (true) {
selector.select();
Set<SelectionKey> selectedKeys = selector.selectedKeys();
Iterator<SelectionKey> iterator = selectedKeys.iterator();
while (iterator.hasNext()) {
SelectionKey key = iterator.next();
iterator.remove();
if (key.isAcceptable()) {
accept(key);
} else if (key.isReadable()) {
read(key);
} else if (key.isWritable()) {
write(key);
}
}
}
}
private void accept(SelectionKey key) throws IOException {
ServerSocketChannel serverSocketChannel = (ServerSocketChannel) key.channel();
SocketChannel socketChannel = serverSocketChannel.accept();
socketChannel.configureBloc
```
0
0
相关推荐
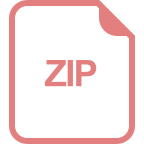
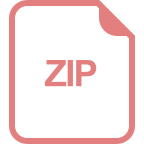





