springboot整合netty,实现socket通信
时间: 2023-06-14 09:01:51 浏览: 83
Spring Boot可以很方便地整合Netty,以实现Socket通信。Netty是一个高可扩展性的Java异步网络编程框架,可以用于开发高性能的网络应用程序和协议服务器。
要实现Spring Boot整合Netty,需要添加Netty依赖。可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.63.Final</version>
</dependency>
```
然后,创建一个NettyServer类,用于启动Netty服务器。在NettyServer类中,可以实现Netty的ChannelInitializer,以初始化服务器的处理程序。例如,可以创建一个EchoServerHandler类,用于处理接收到的消息,并将其回发给客户端。
```java
public class NettyServer {
private final int port;
public NettyServer(int port) {
this.port = port;
}
public void start() throws InterruptedException {
final EchoServerHandler serverHandler = new EchoServerHandler();
EventLoopGroup group = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(group)
.channel(NioServerSocketChannel.class)
.localAddress(new InetSocketAddress(port))
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(serverHandler);
}
});
ChannelFuture f = b.bind().sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully().sync();
}
}
}
```
接下来,可以创建一个Spring Boot应用程序,用于启动Netty服务器。例如,可以添加一个NettyConfiguration类,用于配置并启动Netty服务器。
```java
@Configuration
public class NettyConfiguration {
@Value("${netty.port}")
private int port;
@Bean
public NettyServer nettyServer() {
return new NettyServer(port);
}
@PostConstruct
public void start() throws Exception {
nettyServer().start();
}
}
```
在应用程序的配置文件中可以指定Netty服务器的端口号:
```
netty.port=8888
```
这样,Spring Boot应用程序就可以启动Netty服务器,并实现Socket通信。可以使用Spring Boot自带的WebSocket客户端(例如,Stomp)或其他客户端库(例如,Netty客户端库)与Netty服务器进行通信。
相关推荐
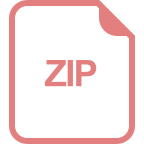
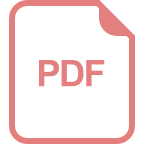














