一个excel数据集在第一次实验特征选择的基础上,进行分类器建模(要求至少使用两种集成学习模型)、评估等过程,分步骤粘贴代码及相应描述。(自行划分训练集与测试集,注意标签样本均衡)
时间: 2024-12-23 18:12:43 浏览: 15
在Excel数据集上进行分类任务,我们需要先将数据加载到Python环境(比如pandas库),然后进行特征选择、划分训练集和测试集,以及使用集成学习模型进行建模和评估。这里是一个简化示例,我们将使用sklearn库,以随机森林和梯度提升机(GBDT)作为集成学习模型,并确保标签样本平衡:
```python
# 导入所需的库
import pandas as pd
from sklearn.model_selection import train_test_split, StratifiedKFold
from sklearn.preprocessing import StandardScaler
from sklearn.ensemble import RandomForestClassifier, GradientBoostingClassifier
from sklearn.metrics import accuracy_score, confusion_matrix
from imblearn.over_sampling import SMOTE
# 加载Excel数据
data = pd.read_excel('your_dataset.xlsx')
# 查看数据前几行
print(data.head())
# 假设label列是目标变量,其他列为特征
X = data.drop('target', axis=1)
y = data['target']
# 处理不平衡标签(假设是SMOTE)
smote = SMOTE()
X_resampled, y_resampled = smote.fit_resample(X, y)
# 划分训练集和测试集(70%训练,30%测试)
X_train, X_test, y_train, y_test = train_test_split(X_resampled, y_resampled, test_size=0.3, random_state=42, stratify=y_resampled)
# 标准化特征
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# 创建并训练集成学习模型
models = [RandomForestClassifier(random_state=42), GradientBoostingClassifier(random_state=42)]
for model in models:
model.fit(X_train, y_train)
# 预测
predictions = [model.predict(X_test) for model in models]
# 评估
accuracy_results = [accuracy_score(y_test, pred) for pred in predictions]
confusion_matrices = [confusion_matrix(y_test, pred) for pred in predictions]
# 输出结果
print(f"Accuracy scores (Random Forest, GBDT): {accuracy_results}")
print("Confusion matrices:")
for i, matrix in enumerate(confusion_matrices):
print(f"\nModel {i + 1}:")
print(matrix)
# 可能的进一步分析:交叉验证,调整参数,AUC-ROC曲线等
```
在这个过程中,我们首先保证了标签样本的均衡,然后分别用随机森林和GBDT进行了建模,最后计算了各自模型的准确率和混淆矩阵来进行性能评估。如果数据量大或需要更高级的分析,可以考虑使用交叉验证进行模型选择和超参数优化。
阅读全文
相关推荐
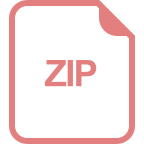
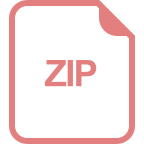
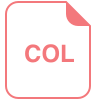
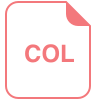
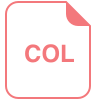
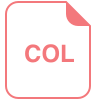
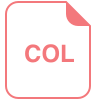
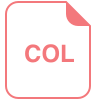
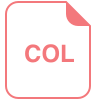
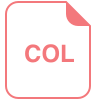
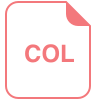
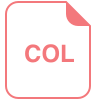
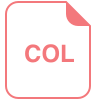
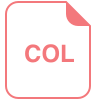
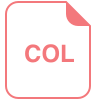
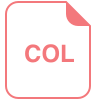
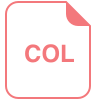
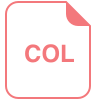