坐标系两点的距离c语言
时间: 2024-09-13 08:18:45 浏览: 74
在C语言中计算二维坐标系中两点之间的距离,通常采用欧几里得距离公式,也称为勾股定理。对于两个点 (x1, y1) 和 (x2, y2),距离d可以按照下面的公式计算:
```c
double distance(double x1, double y1, double x2, double y2) {
return sqrt((x2 - x1) * (x2 - x1) + (y2 - y1) * (y2 - y1));
}
```
在这个函数中,`sqrt()` 函数用于计算平方根,`*` 表示乘法。这个函数接受四个参数,分别代表两个点的横纵坐标,并返回它们之间的距离。
如果你想计算三维空间(x, y, z)中的两点距离,只需要将公式稍作调整即可:
```c
double distance3D(double x1, double y1, double z1, double x2, double y2, double z2) {
return sqrt((x2 - x1) * (x2 - x1) + (y2 - y1) * (y2 - y1) + (z2 - z1) * (z2 - z1));
}
```
相关问题
c语言极坐标求两点距离
极坐标是一种二维坐标系,其中每个点由极径和极角确定。在极坐标系中,两点之间的距离可以通过它们的极坐标来计算。具体而言,设两点的极坐标分别为(r1,θ1)和(r2,θ2),则它们之间的距离d可以通过以下公式计算:
d = sqrt(r1^2 + r2^2 - 2*r1*r2*cos(θ1-θ2))
下面是一个C语言程序,用于计算两个点的极坐标并计算它们之间的距离:
```c
#include <stdio.h>
#include <math.h>
int main() {
float r1, r2, theta1, theta2, x1, x2, y1, y2, distance;
printf("Enter the polar coordinates of point A (r, theta): ");
scanf("%f, %f", &r1, &theta1);
printf("Enter the polar coordinates of point B (r, theta): ");
scanf("%f, %f", &r2, &theta2);
x1 = r1 * cos(theta1);
y1 = r1 * sin(theta1);
x2 = r2 * cos(theta2);
y2 = r2 * sin(theta2);
distance = sqrt(pow((x1-x2),2) + pow((y1-y2),2));
printf("The distance between the two points is: %.2f", distance);
return 0;
}
```
该程序首先要求用户输入两个点的极坐标,然后将它们转换为直角坐标系中的坐标。最后,程序使用上述公式计算两点之间的距离,并将结果输出到屏幕上。
输人两组数据x1、yī 和x:y,分别代表平面直角坐标系中的两个点,求此两点间的距离。 c语言
在C语言中,计算两个二维坐标点(x1, y1)和(x2, y2)之间的欧几里得距离可以使用下面的公式:
```c
#include <math.h> // 包含数学库,用于sqrt函数
double distance(double x1, double y1, double x2, double y2) {
// 欧氏距离公式: sqrt((x2-x1)^2 + (y2-y1)^2)
double dx = x2 - x1;
double dy = y2 - y1;
return sqrt(dx * dx + dy * dy);
}
// 如果给定的是x1, y1, x:y的形式,需要先解析输入
void parse_coordinates(char* input, double* x1, double* y1) {
char* delimiter = ":";
char* point = strtok(input, delimiter);
*x1 = atof(point); // 将字符串转换为浮点数
point = strtok(NULL, delimiter);
*y1 = atof(point);
}
int main() {
double x1, y1, x2, y2;
char coordinates[] = "x1:y1, x2:y2"; // 示例输入
parse_coordinates(coordinates, &x1, &y1); // 解析第一个点
parse_coordinates(strtok(NULL, ","), &x2, &y2); // 解析第二个点
double dist = distance(x1, y1, x2, y2);
printf("两点间距离为: %.2f\n", dist);
return 0;
}
```
在这个示例中,`parse_coordinates`函数负责将输入的坐标字符串分割成独立的数值,并将其赋值给对应的变量。`distance`函数则计算并返回两点之间的距离。
阅读全文
相关推荐
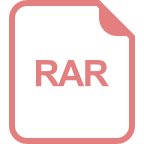
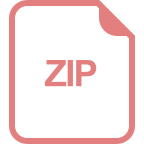
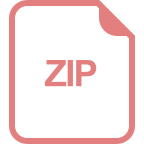
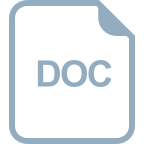
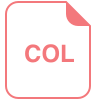
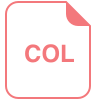










