写一个unity Input Manager详细代码
时间: 2023-08-31 17:25:15 浏览: 103
### 回答1:
下面是一份 Unity Input Manager 的代码示例:
```
using UnityEngine;
public class InputManager : MonoBehaviour
{
public string horizontalAxis = "Horizontal";
public string verticalAxis = "Vertical";
public string jumpButton = "Jump";
public string fireButton = "Fire1";
private void Update()
{
float horizontal = Input.GetAxis(horizontalAxis);
float vertical = Input.GetAxis(verticalAxis);
if (Input.GetButtonDown(jumpButton))
{
// 处理跳跃事件
}
if (Input.GetButtonDown(fireButton))
{
// 处理开火事件
}
}
}
```
在这份代码中,我们定义了四个字符串变量:`horizontalAxis`,`verticalAxis`,`jumpButton` 和 `fireButton`。这些变量代表着游戏中的四个操作,即左右移动,上下移动,跳跃和开火。
在 `Update` 函数中,我们使用 `Input.GetAxis` 函数获取了横向和纵向轴的值,并使用 `Input.GetButtonDown` 函数处理跳跃和开火事件。
请注意,这份代码仅是一个简单的示例,它可以作为您自己代码的基础。您可以在此基础上添加自己的代码,以实现更复杂的操作。
### 回答2:
Unity中的Input Manager是一个用于管理用户输入的工具,可以通过它来获取玩家的键盘、鼠标或者手柄输入。下面是一个简单的Unity Input Manager的代码示例:
```csharp
using UnityEngine;
public class InputManager : MonoBehaviour
{
public float moveSpeed = 5f;
private Vector3 moveDirection;
private void Update()
{
// 获取用户输入的水平和垂直轴的值
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
// 设置移动方向
moveDirection = new Vector3(horizontalInput, 0, verticalInput).normalized;
// 移动游戏对象
transform.Translate(moveDirection * moveSpeed * Time.deltaTime);
}
}
```
在上述代码中,我们创建了一个名为"InputManager"的MonoBehaviour类。在Update方法中,我们使用了`Input.GetAxis`函数来获取玩家输入的水平和垂直轴的值,这里的"Horizontal"和"Vertical"是Unity内置的输入管理器定义的默认轴。获取到用户的输入后,我们使用`normalized`函数将输入向量归一化,保证每次移动长度为1,并设置了移动速度`moveSpeed`来调整移动距离。最后,我们使用`Translate`函数将游戏对象按照移动方向和速度进行移动。
上述代码是一个简单的Unity Input Manager代码示例,可以用来获取用户的输入并进行相应的移动操作。根据实际需求,你还可以扩展该代码,例如:添加更多的输入处理逻辑、处理不同平台的输入方式等。
### 回答3:
Unity的Input Manager是一个用于管理输入的系统,用于处理玩家的输入设备,如键盘、鼠标和手柄。下面是一个基本的Unity Input Manager的示例代码。
1. 首先,需要创建一个新的C#脚本,命名为"InputManager"。
2. 在该脚本中,需要使用Unity的命名空间`UnityEngine`和`UnityEngine.InputSystem`。
3. 在脚本中添加以下成员变量:
```
private PlayerInput _playerInput;
private float _horizontalInput;
private float _verticalInput;
private bool _jumpInput;
```
4. 在`Start`方法中,获取`PlayerInput`组件并将其赋值给`_playerInput`变量:
```
private void Start()
{
_playerInput = GetComponent<PlayerInput>();
}
```
5. 实现`Update`方法以获取玩家的输入:
```
private void Update()
{
_horizontalInput = _playerInput.actions["Move Horizontal"].ReadValue<float>();
_verticalInput = _playerInput.actions["Move Vertical"].ReadValue<float>();
_jumpInput = _playerInput.actions["Jump"].ReadValue<float>() > 0;
}
```
6. 在这个例子中,假设已经在Input Manager中设置了名称为"Move Horizontal"和"Move Vertical"的两个输入轴,以及一个名称为"Jump"的输入按钮。
7. 在脚本中可以通过`_horizontalInput`和`_verticalInput`来获取水平和垂直输入的值,在需要使用时可以直接访问这些变量。
8. 同样,可以通过`_jumpInput`来判断玩家是否按下了跳跃按钮。
9. 在场景中的游戏对象上添加该脚本。
以上就是一个基本的Unity Input Manager的示例代码,可以通过这种方式来管理输入设备和获取玩家的输入。当然,实际使用时还可以根据需要进行进一步的扩展和优化。
阅读全文
相关推荐
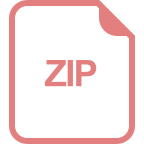
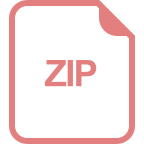
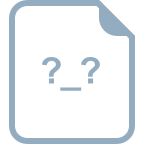
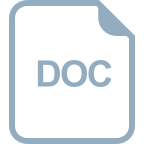




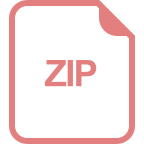








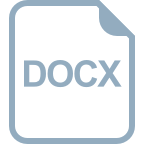
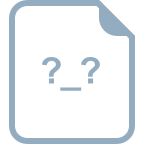