并行串行超线程计算圆周率
时间: 2023-10-17 21:05:12 浏览: 88
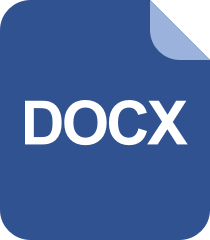
软件构造原理作业 lab03
计算圆周率是一个计算密集型任务,可以使用并行计算和超线程来提高计算效率。下面分别介绍并行计算、串行计算和超线程计算的方法。
1. 并行计算
并行计算是指将任务划分为多个子任务,每个子任务在不同的处理器上并行执行,最终将所有子任务的结果合并得到最终结果。在计算圆周率的过程中,可以将生成随机点的任务划分为多个子任务,每个子任务在不同的线程中并行执行。最后,将所有子任务的结果合并,得到最终的圆周率值。
下面是一个使用 Python 进行并行计算的例子:
```python
import random
from multiprocessing import Pool
def generate_points(n):
points_inside_circle = 0
for i in range(n):
x, y = random.uniform(-1, 1), random.uniform(-1, 1)
if x**2 + y**2 <= 1:
points_inside_circle += 1
return points_inside_circle
def estimate_pi_parallel(num_tasks, num_points_per_task):
pool = Pool(processes=num_tasks)
num_points_inside_circle = sum(pool.map(generate_points, [num_points_per_task] * num_tasks))
return 4 * num_points_inside_circle / (num_tasks * num_points_per_task)
print(estimate_pi_parallel(4, 1000000))
```
这个例子中,我们将任务划分为 4 个子任务,在 4 个进程中并行执行。每个子任务生成 1000000 个随机点,统计落在内切圆内的点数,并返回最终的圆周率值。使用 `multiprocessing.Pool` 可以方便地创建进程池,并通过 `map` 方法将任务分配到不同的进程中执行。
2. 串行计算
串行计算是指将任务顺序执行,每个任务执行完毕后再执行下一个任务。在计算圆周率的过程中,可以顺序生成随机点,统计落在内切圆内的点数,最后计算圆周率值。
下面是一个使用 Python 进行串行计算的例子:
```python
import random
def estimate_pi_serial(num_points):
num_points_inside_circle = 0
for i in range(num_points):
x, y = random.uniform(-1, 1), random.uniform(-1, 1)
if x**2 + y**2 <= 1:
num_points_inside_circle += 1
return 4 * num_points_inside_circle / num_points
print(estimate_pi_serial(4000000))
```
这个例子中,我们顺序生成 4000000 个随机点,统计落在内切圆内的点数,并返回最终的圆周率值。
3. 超线程计算
超线程是指在单个物理处理器上模拟出多个逻辑处理器,从而提高计算效率。在计算圆周率的过程中,可以利用超线程将多个线程调度到单个物理处理器上执行,从而提高计算效率。
下面是一个使用 Python 进行超线程计算的例子:
```python
import random
import threading
class GeneratePointsThread(threading.Thread):
def __init__(self, num_points):
super().__init__()
self.num_points = num_points
self.num_points_inside_circle = 0
def run(self):
for i in range(self.num_points):
x, y = random.uniform(-1, 1), random.uniform(-1, 1)
if x**2 + y**2 <= 1:
self.num_points_inside_circle += 1
def estimate_pi_hyperthreading(num_threads, num_points_per_thread):
threads = [GeneratePointsThread(num_points_per_thread) for i in range(num_threads)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
num_points_inside_circle = sum([thread.num_points_inside_circle for thread in threads])
return 4 * num_points_inside_circle / (num_threads * num_points_per_thread)
print(estimate_pi_hyperthreading(4, 1000000))
```
这个例子中,我们创建 4 个线程,每个线程生成 1000000 个随机点,统计落在内切圆内的点数。使用 `threading.Thread` 可以方便地创建线程,并通过 `start` 和 `join` 方法启动和等待线程执行完毕。最后,将所有线程的结果合并,得到最终的圆周率值。
阅读全文
相关推荐
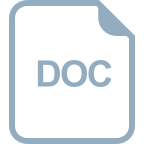
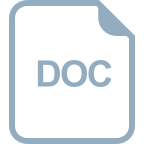
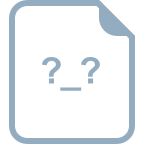





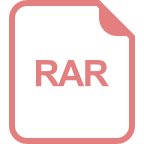
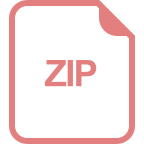
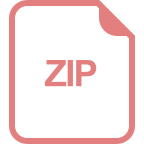
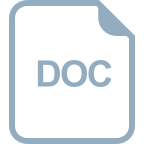
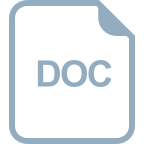
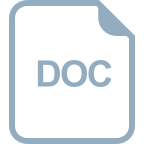
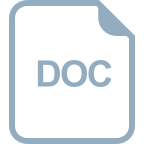