使用python根据给定数据,绘制物流公司物流费用统计堆积面积图
时间: 2024-03-05 22:47:34 浏览: 27
好的,您需要先安装matplotlib库,可以使用以下命令进行安装:
```
pip install matplotlib
```
然后,您可以使用以下代码绘制物流公司物流费用统计堆积面积图:
```python
import matplotlib.pyplot as plt
# 物流公司列表
companies = ['顺丰', '圆通', '韵达', '中通', '申通']
# 物流费用数据,每个元素为一个列表,表示每个物流公司的费用统计
costs = [[300, 500, 700, 900, 1100],
[200, 400, 600, 800, 1000],
[100, 300, 500, 700, 900],
[400, 600, 800, 1000, 1200],
[500, 700, 900, 1100, 1300]]
# 绘制堆积面积图
plt.stackplot(range(1, 6), costs, labels=companies)
# 添加标题和坐标轴标签
plt.title('物流公司物流费用统计')
plt.xlabel('月份')
plt.ylabel('费用(元)')
# 添加图例
plt.legend(loc='upper left')
# 显示图形
plt.show()
```
运行上述代码,即可得到物流公司物流费用统计堆积面积图。您可以根据自己的数据进行修改。
相关问题
围成面积python
要计算围成的面积,可以使用两种方法。
方法一:
首先,需要导入matplotlib.pyplot、pandas和shapely.geometry库。
然后,定义纵坐标y和横坐标x。
接下来,将x和y合并为点列表,并绘制散点图。
然后,使用双指针法计算相邻两点围成的面积累加和。
最后,输出相邻两点围成的面积累加和。
方法二:
给定一个整数列表nums。
使用双指针法进行计算,初始化右指针为数组长度减一,左指针为0,面积S为0。
右指针不断向左移动,左指针不断向右移动,当右指针和左指针相遇时中断。
在每次移动指针时,比较当前指针指向的元素大小,移动较小数字对应的指针,并更新最大面积S。
最后,输出最大的面积S。
所以,对于给定的python代码和问题,围成面积的计算可以通过以上两种方法进行。
逻辑斯蒂分布绘制分布图
### 回答1:
要绘制逻辑斯蒂分布的分布图,可以使用 Matplotlib 库。下面是一个简单的例子,展示如何绘制逻辑斯蒂分布的概率密度函数:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import logistic
mu = 0
sigma = 1
x = np.linspace(-10, 10, 1000)
pdf = logistic.pdf((x - mu) / sigma)
plt.plot(x, pdf, label='Logistic Distribution')
plt.legend()
plt.show()
```
这段代码将会生成一个逻辑斯蒂分布的概率密度函数图像,其中 x 轴表示自变量,y 轴表示概率密度函数值。你可以根据需要调整参数,比如均值、标准差、自变量的范围等,来绘制出你需要的逻辑斯蒂分布图像。
### 回答2:
逻辑斯蒂分布(Logistic distribution)是一种常见的概率分布,其概率密度函数具有S形曲线。为了绘制逻辑斯蒂分布的分布图,可以按照以下步骤进行:
1. 确定逻辑斯蒂分布的参数:逻辑斯蒂分布由两个参数决定,即位置参数μ和形状参数σ。根据具体情况,选择合适的参数值。
2. 计算概率密度函数(PDF):根据逻辑斯蒂分布的概率密度函数公式,计算在给定参数下的概率密度值。逻辑斯蒂分布的PDF公式为:
f(x) = (1 / (σ * sqrt(2π))) * exp(-(x-μ) / σ) / (1 + exp(-(x-μ) / σ))^2
3. 设定x轴范围:确定需要绘制概率密度函数的x轴范围。根据实际情况确定范围,如[-10, 10]或[0, 1]等。
4. 绘制概率密度函数图形:使用统计软件或编程语言,利用计算出的概率密度函数进行绘图。绘制图形时,将x轴范围划分成一定数量的点,计算每个点对应的概率密度值,并在图中以连续曲线的形式表示。
5. 添加坐标轴和标题:在绘制的图形中添加x轴、y轴的标签,以及适当的标题,以便理解图形的含义。
通过以上步骤,我们可以绘制出逻辑斯蒂分布的分布图。通过观察分布图的形状和特征,我们可以对逻辑斯蒂分布进行更深入的理解。
### 回答3:
逻辑斯蒂分布是一种常用于二元分类问题的概率分布模型。其概率密度函数可以表示为:
f(x)= e^(-x) / (1+e^(-x))^2
其中,x是自变量,f(x)是概率密度函数。逻辑斯蒂分布的概率密度函数是一个S形曲线,其特点是在x趋近正无穷时,f(x)趋近于1,在x趋近负无穷时,f(x)趋近于0。
为了绘制逻辑斯蒂分布的分布图,我们需要选取一个适当的x范围,然后根据概率密度函数计算对应的概率密度值,最后将这些点连线得到曲线图。
首先,选择一个适当的x范围,例如取x从-6到6之间的一系列数值,每隔0.1取一个值。这样我们有61个x值。
然后,根据逻辑斯蒂分布的概率密度函数,计算每个x对应的概率密度值。将x代入概率密度函数中,得到相应的概率密度值。
最后,将这些点按照顺序连线,就得到了逻辑斯蒂分布的分布图。
在该分布图中,x轴表示自变量x的取值范围,y轴表示对应的概率密度值。随着x值的变化,对应的概率密度值在S形曲线上变化。
逻辑斯蒂分布的曲线通常有一个对数几率的解释,即在某个x值上,分布曲线上方的面积表示对应的事件发生的概率,而曲线下方的面积表示对应的事件不发生的概率。这样,我们可以通过观察分布图来理解逻辑斯蒂分布的特性和应用。
相关推荐
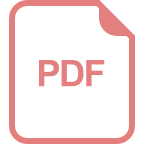
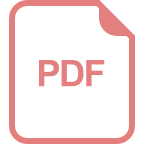
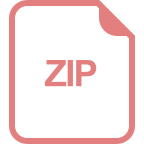












