sklearn调用逻辑回归模块,自编程实现逻辑回归算法复杂的完整代码
时间: 2023-10-13 17:09:38 浏览: 100
好的,以下是一个简单的逻辑回归算法的Python代码实现,使用了sklearn库中的LogisticRegression模块作为参考:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义sigmoid函数
def sigmoid(z):
return 1 / (1 + np.exp(-z))
# 定义逻辑回归模型
class LogisticRegression:
def __init__(self, learning_rate=0.01, num_iterations=100):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
self.weights = None
self.bias = None
def fit(self, X, y):
# 初始化参数
m, n = X.shape
self.weights = np.zeros((n, 1))
self.bias = 0
# 梯度下降
for i in range(self.num_iterations):
z = np.dot(X, self.weights) + self.bias
A = sigmoid(z)
cost = -1/m * np.sum(y*np.log(A) + (1-y)*np.log(1-A))
dz = A - y
dw = 1/m * np.dot(X.T, dz)
db = 1/m * np.sum(dz)
self.weights -= self.learning_rate * dw
self.bias -= self.learning_rate * db
if i % 1000 == 0:
print("Cost after iteration %i: %f" % (i, cost))
def predict(self, X):
z = np.dot(X, self.weights) + self.bias
A = sigmoid(z)
y_pred = np.round(A)
return y_pred
# 测试
np.random.seed(0)
X = np.random.randn(100, 2)
y = np.random.randint(0, 2, size=(100, 1))
model = LogisticRegression(learning_rate=0.1, num_iterations=10000)
model.fit(X, y)
y_pred = model.predict(X)
plt.scatter(X[:, 0], X[:, 1], c=y_pred)
plt.show()
```
这段代码实现了一个简单的逻辑回归算法,包括sigmoid函数、梯度下降、模型拟合和预测等步骤。可以通过调整learning_rate和num_iterations参数来优化模型的性能。
阅读全文
相关推荐
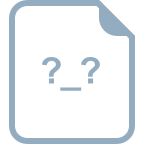




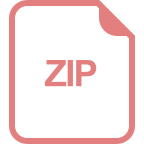
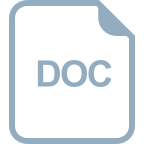
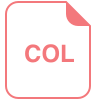
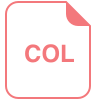
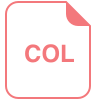
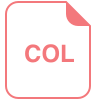
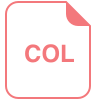
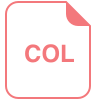
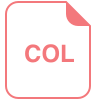
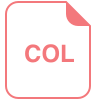
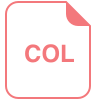
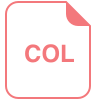