sklearn调用逻辑回归模块,自编程实现逻辑回归算法代码
时间: 2023-10-13 10:13:06 浏览: 100
好的,下面是自编程实现逻辑回归算法的一份Python代码:
```python
import numpy as np
class MyLogisticRegression:
def __init__(self, learning_rate=0.01, num_iterations=10000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def cost_function(self, X, y, theta):
m = len(y)
h = self.sigmoid(np.dot(X, theta))
J = -1/m * np.sum(y * np.log(h) + (1-y) * np.log(1-h))
return J
def gradient_descent(self, X, y, theta):
m = len(y)
for i in range(self.num_iterations):
h = self.sigmoid(np.dot(X, theta))
gradient = np.dot(X.T, (h - y)) / m
theta -= self.learning_rate * gradient
return theta
def fit(self, X, y):
X = np.insert(X, 0, 1, axis=1) # add bias term
n = X.shape[1]
self.theta = np.zeros((n,1))
self.theta = self.gradient_descent(X, y, self.theta)
def predict(self, X):
X = np.insert(X, 0, 1, axis=1) # add bias term
y_pred = np.round(self.sigmoid(np.dot(X, self.theta)))
return y_pred
```
这里使用了梯度下降算法求解逻辑回归模型,其中`sigmoid`函数用于计算逻辑回归模型的预测值,`cost_function`函数用于计算模型的损失函数,`gradient_descent`函数用于求解模型参数,`fit`函数用于训练模型,`predict`函数用于对新数据进行预测。
阅读全文
相关推荐
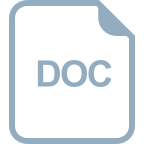
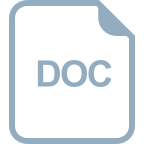
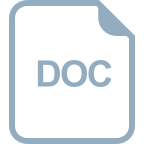




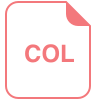
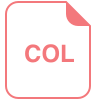
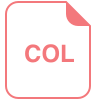
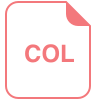
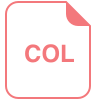
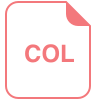
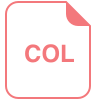
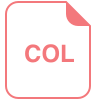
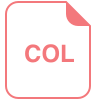
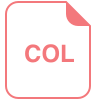
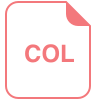
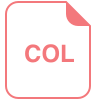