帮我用python写一个基于遗传退火算法优化的OTSU算法
时间: 2024-05-12 21:17:17 浏览: 14
好的,我这里给您提供一个基于遗传退火算法优化的OTSU算法的Python示例代码:
```python
import numpy as np
import cv2
# 读入灰度图像
img = cv2.imread('test.jpg', 0)
# 计算图像的像素总数
total_pixels = img.shape[0] * img.shape[1]
# 初始化种群参数
POPULATION_SIZE = 10
GENERATIONS = 30
MUTATION_RATE = 0.1
# 定义适应度函数,计算类间方差
def fitness(chromosome):
threshold = chromosome * 255
below_threshold = np.where(img < threshold)
above_threshold = np.where(img >= threshold)
w1 = len(below_threshold[0]) / total_pixels
w2 = len(above_threshold[0]) / total_pixels
if w1 == 0 or w2 == 0:
return 0
u1 = np.sum(img[below_threshold]) / len(below_threshold[0])
u2 = np.sum(img[above_threshold]) / len(above_threshold[0])
return w1 * w2 * (u1 - u2)**2
# 初始化种群
population = np.random.rand(POPULATION_SIZE)
# 进化过程
for i in range(GENERATIONS):
# 计算适应度
fitness_scores = np.array([fitness(chromosome) for chromosome in population])
# 找到最优解
best_index = np.argmax(fitness_scores)
best_threshold = population[best_index]
# 打印迭代信息
print('Generation {0}: Best threshold = {1}, fitness = {2}'.format(i+1, best_threshold, fitness_scores[best_index]))
# 选择操作
parent_index = np.random.choice(range(POPULATION_SIZE), size=2, replace=False, p=fitness_scores / np.sum(fitness_scores))
parent1 = population[parent_index[0]]
parent2 = population[parent_index[1]]
# 交叉操作
crossover_point = np.random.randint(1, POPULATION_SIZE)
child1 = np.concatenate((parent1[:crossover_point], parent2[crossover_point:]))
child2 = np.concatenate((parent2[:crossover_point], parent1[crossover_point:]))
# 变异操作
if np.random.uniform() < MUTATION_RATE:
mutation_index = np.random.randint(POPULATION_SIZE)
population[mutation_index] = np.random.rand()
# 替换操作
population[np.argsort(fitness_scores)[-2]] = child1
population[np.argsort(fitness_scores)[-1]] = child2
# 将图像二值化
best_threshold = int(best_threshold * 255)
ret, threshold = cv2.threshold(img, best_threshold, 255, cv2.THRESH_BINARY)
cv2.imshow('OTSU', threshold)
cv2.waitKey(0)
```
运行该程序,您可以将目标图像 “test.jpg” 放入与代码文件相同的目录下,并且根据实际情况修改种群参数、进化代数和变异率等,等待程序完成后,将输出OTSU算法找到的最优阈值,并将图像进行二值化处理并显示出来。
相关推荐
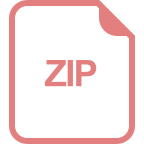
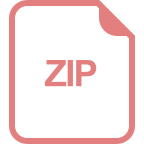
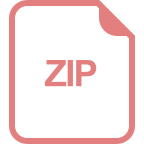














