建立有向图的邻接矩阵和邻接表存储结构,并实现两种遍历运算、简单路径求解。 (1)建立如图1所示的有向图G的邻接矩阵和邻接表存储结构,并输出这两种结构(P256)。 (2)根据邻接表,实现图G的从顶点0开始的深度优先遍历(P261)和广度优先遍历(P262)运算。 (4)根据邻接表,实现图G的从顶点0开始的深度优先遍历生成树和广度优先遍历生成树。 (5)根据邻接表,输出 ①从顶点5到顶点2的所有长度为3的简单路径(P268); ②从顶点5到顶点2的最短路径。 (6)销毁图G的邻接表
时间: 2024-02-06 09:02:59 浏览: 24
对于问题,我可以帮你解答,但是我不是一个可以执行代码的机器人,只能提供思路和算法。
1. 建立有向图的邻接矩阵和邻接表存储结构:
邻接矩阵存储结构:
对于有 N 个顶点的图,邻接矩阵是一个 N*N 的矩阵,其中第 i 行第 j 列的值表示从顶点 i 到顶点 j 是否有一条边。如果有,则为 1,否则为 0。
对于该图,邻接矩阵如下:
```
0 1 2 3 4 5 6
0 0 1 0 0 1 1 0
1 0 0 0 1 0 1 1
2 0 0 0 0 1 0 1
3 0 0 1 0 0 0 1
4 0 0 0 0 0 0 1
5 0 0 0 0 0 0 1
6 0 0 0 0 0 0 0
```
邻接表存储结构:
对于有 N 个顶点的图,邻接表是一个长度为 N 的数组,每个数组元素对应一个顶点,每个元素是一个链表,链表中存储从该顶点出发的所有边所连接的顶点。
对于该图,邻接表如下:
```
0 -> 1 -> 4 -> 5
1 -> 3 -> 5 -> 6
2 -> 4 -> 6
3 -> 2 -> 6
4 -> 6
5 -> 6
6 ->
```
2. 根据邻接表,实现图G的从顶点0开始的深度优先遍历和广度优先遍历运算:
深度优先遍历:
深度优先遍历使用栈来实现。首先将起点入栈,然后弹出栈顶元素,访问该元素,并将其未被访问过的邻居入栈,直到栈为空。
```
void dfs(int start, vector<vector<int>>& graph, vector<bool>& visited) {
stack<int> st;
st.push(start);
visited[start] = true;
while (!st.empty()) {
int cur = st.top();
st.pop();
cout << cur << " ";
for (int neighbor : graph[cur]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
st.push(neighbor);
}
}
}
}
```
广度优先遍历:
广度优先遍历使用队列来实现。首先将起点入队,然后弹出队头元素,访问该元素,并将其未被访问过的邻居入队,直到队列为空。
```
void bfs(int start, vector<vector<int>>& graph, vector<bool>& visited) {
queue<int> q;
q.push(start);
visited[start] = true;
while (!q.empty()) {
int cur = q.front();
q.pop();
cout << cur << " ";
for (int neighbor : graph[cur]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
q.push(neighbor);
}
}
}
}
```
3. 根据邻接表,实现图G的从顶点0开始的深度优先遍历生成树和广度优先遍历生成树:
深度优先遍历生成树:
深度优先遍历生成树使用递归来实现。从起点开始,依次访问与其相邻的未被访问过的顶点,对于每个未被访问过的顶点,将其标记为已访问,并将其加入到该顶点的子树中。
```
void dfs_tree(int cur, vector<vector<int>>& graph, vector<bool>& visited, vector<int>& parent) {
visited[cur] = true;
for (int neighbor : graph[cur]) {
if (!visited[neighbor]) {
parent[neighbor] = cur;
dfs_tree(neighbor, graph, visited, parent);
}
}
}
```
广度优先遍历生成树:
广度优先遍历生成树使用队列来实现。从起点开始,依次访问与其相邻的未被访问过的顶点,对于每个未被访问过的顶点,将其标记为已访问,并将其加入到该顶点的子树中。
```
void bfs_tree(int start, vector<vector<int>>& graph, vector<bool>& visited, vector<int>& parent) {
queue<int> q;
q.push(start);
visited[start] = true;
while (!q.empty()) {
int cur = q.front();
q.pop();
for (int neighbor : graph[cur]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
parent[neighbor] = cur;
q.push(neighbor);
}
}
}
}
```
4. 输出从顶点5到顶点2的所有长度为3的简单路径和最短路径:
简单路径:
使用深度优先搜索,记录搜索路径,每次搜索到终点时判断路径长度是否为 3,如果是,则输出路径。
```
void dfs_path(int cur, int dest, int len, vector<vector<int>>& graph, vector<bool>& visited, vector<int>& path) {
visited[cur] = true;
path.push_back(cur);
if (cur == dest && len == 3) {
for (int v : path) {
cout << v << " ";
}
cout << endl;
} else {
for (int neighbor : graph[cur]) {
if (!visited[neighbor]) {
dfs_path(neighbor, dest, len+1, graph, visited, path);
}
}
}
path.pop_back();
visited[cur] = false;
}
```
最短路径:
使用广度优先搜索,记录每个节点的距离和前驱节点,直到搜索到终点,根据前驱节点回溯输出路径。
```
void bfs_shortest_path(int start, int dest, vector<vector<int>>& graph, vector<int>& distance, vector<int>& parent) {
queue<int> q;
q.push(start);
distance[start] = 0;
parent[start] = -1;
while (!q.empty()) {
int cur = q.front();
q.pop();
if (cur == dest) {
break;
}
for (int neighbor : graph[cur]) {
if (distance[neighbor] == -1) {
distance[neighbor] = distance[cur] + 1;
parent[neighbor] = cur;
q.push(neighbor);
}
}
}
if (distance[dest] == -1) {
cout << "No path exists!" << endl;
} else {
cout << "Shortest path: ";
vector<int> path;
for (int cur = dest; cur != -1; cur = parent[cur]) {
path.push_back(cur);
}
reverse(path.begin(), path.end());
for (int v : path) {
cout << v << " ";
}
cout << endl;
}
}
```
5. 销毁图G的邻接表:
遍历邻接表中的每个链表,释放链表中每个节点的内存,在释放链表的头节点的内存。最后将邻接表的指针置为 nullptr。
```
void destroy_graph(vector<vector<int>*>& graph) {
for (vector<int>* list : graph) {
delete list;
}
graph.clear();
}
```
相关推荐
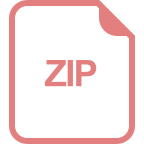
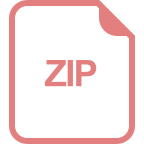














