用c语言从键盘输入一段表达式(字符串),其中含有整数、加、减、乘、除并计算表达式的值
时间: 2023-08-16 12:04:42 浏览: 93
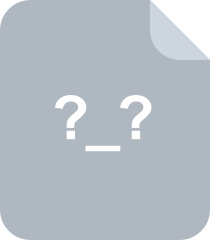
c语言算术表达式,加减乘除、乘方、括号。依次输出在求值过程中运算数栈内的栈顶数据变化过程,并最终输出表达式的值

好的,我可以帮你解决这个问题。首先,我们需要将输入的字符串转换成可以计算的表达式。这个过程需要使用到栈和逆波兰表达式的算法。
以下是代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define STACK_SIZE 100
typedef struct {
int top;
char data[STACK_SIZE];
} Stack;
void stack_init(Stack *s) {
s->top = -1;
}
int stack_is_empty(Stack *s) {
return s->top == -1;
}
int stack_is_full(Stack *s) {
return s->top == STACK_SIZE - 1;
}
void stack_push(Stack *s, char c) {
if (stack_is_full(s)) {
printf("Stack overflow!\n");
exit(1);
}
s->data[++s->top] = c;
}
char stack_pop(Stack *s) {
if (stack_is_empty(s)) {
printf("Stack underflow!\n");
exit(1);
}
return s->data[s->top--];
}
int stack_top(Stack *s) {
if (stack_is_empty(s)) {
printf("Stack underflow!\n");
exit(1);
}
return s->data[s->top];
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int get_operator_priority(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
void infix_to_postfix(char *infix_expression, char *postfix_expression) {
Stack operator_stack;
stack_init(&operator_stack);
char *p = infix_expression;
char *q = postfix_expression;
while (*p != '\0') {
if (isdigit(*p)) {
*q++ = *p++;
while (isdigit(*p)) {
*q++ = *p++;
}
*q++ = ' ';
} else if (is_operator(*p)) {
while (!stack_is_empty(&operator_stack) && is_operator(stack_top(&operator_stack)) && get_operator_priority(stack_top(&operator_stack)) >= get_operator_priority(*p)) {
*q++ = stack_pop(&operator_stack);
*q++ = ' ';
}
stack_push(&operator_stack, *p++);
} else if (*p == '(') {
stack_push(&operator_stack, *p++);
} else if (*p == ')') {
while (stack_top(&operator_stack) != '(') {
*q++ = stack_pop(&operator_stack);
*q++ = ' ';
}
stack_pop(&operator_stack);
p++;
} else {
p++;
}
}
while (!stack_is_empty(&operator_stack)) {
*q++ = stack_pop(&operator_stack);
*q++ = ' ';
}
*q = '\0';
}
int calculate_postfix_expression(char *postfix_expression) {
Stack operand_stack;
stack_init(&operand_stack);
char *p = postfix_expression;
while (*p != '\0') {
if (isdigit(*p)) {
int num = 0;
while (isdigit(*p)) {
num = num * 10 + (*p - '0');
p++;
}
stack_push(&operand_stack, num);
} else if (is_operator(*p)) {
int op1, op2;
op2 = stack_pop(&operand_stack);
op1 = stack_pop(&operand_stack);
switch (*p) {
case '+':
stack_push(&operand_stack, op1 + op2);
break;
case '-':
stack_push(&operand_stack, op1 - op2);
break;
case '*':
stack_push(&operand_stack, op1 * op2);
break;
case '/':
stack_push(&operand_stack, op1 / op2);
break;
default:
printf("Invalid operator!\n");
exit(1);
}
p++;
} else if (*p == ' ') {
p++;
} else {
printf("Invalid character!\n");
exit(1);
}
}
return stack_pop(&operand_stack);
}
int main() {
char infix_expression[100];
char postfix_expression[100];
printf("Enter an infix expression: ");
fgets(infix_expression, sizeof(infix_expression), stdin);
infix_to_postfix(infix_expression, postfix_expression);
printf("Postfix expression: %s\n", postfix_expression);
int result = calculate_postfix_expression(postfix_expression);
printf("Result: %d\n", result);
return 0;
}
```
在这个示例代码中,我们定义了一个栈的结构体和一些基本的栈操作函数。在 `infix_to_postfix` 函数中,我们使用了栈和逆波兰表达式的算法将中缀表达式转换为后缀表达式。在 `calculate_postfix_expression` 函数中,我们使用了栈来计算后缀表达式的值。
你可以通过这些函数来实现从键盘输入一个表达式并计算它的值。
阅读全文
相关推荐
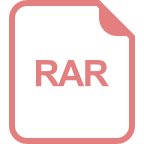





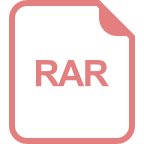
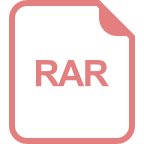
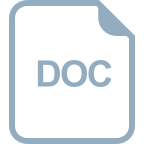
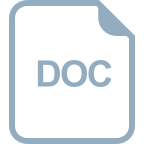
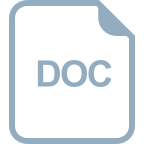
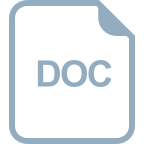
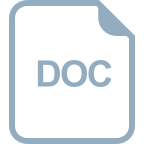
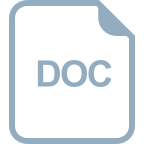
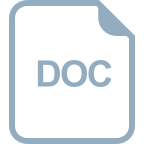
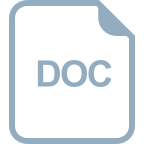